To implement solid programming principles, you must start by understanding the very foundation on which they are based. Sometimes I think of object-oriented programming as building a complex structure: the foundations must be strong for the entire construction to stand stably. Among these foundations, the Interface Segregation Principle stands out, marked by the letter I in the acronym SOLID. But what does it actually mean and why is it so important? Get ready for a journey through the twists and turns of coding!
The interface segregation principle states that clients should not be forced to depend on interfaces they do not use. Sounds wise, right? Imagine you are ordering a pizza, and the restaurant forces you to review each ingredient of the dish – from the dough to the olives. Of course, you don’t want to rate olives when you’re not ordering them! And that’s exactly the point: every external dependency should be minimized to the bare minimum. This not only makes life easier for developers but also makes the code more flexible and easier to maintain.
In practice, this means that instead of having one huge interface that is responsible for everything – let’s say it provides methods to:
- retrieve data
- store data
- delete data
it’s better to create several smaller, more specialized interfaces. Like in a well-organized library, where each department is handled by the appropriate staff member. Isn’t that logical? The psychology behind this is clear: the more complex a system, the harder it is to navigate, which leads to more errors and misunderstandings.
Let’s not forget that the I principle in SOLID does not exist in a vacuum. It is part of a larger set of practices aimed at improving the quality and specialization of your code. Proper implementation of the interface segregation principle can:
- improve the flexibility of systems
- increase understanding of what each interface actually does
As a result, this reduces the risk of accidental changes that can lead to serious problems throughout the application architecture.
In the next part of the article, we will take a look at specific examples that will help you better understand not only the theory but also the real-world application of the Interface Segregation Principle. After all, code is not just a collection of lines of text – it’s a story you tell the programming world. Are you ready to put the I principle into practice and make your code more elegant and understandable?
Surely you've encountered an interface that was more chaotic than maps in a labyrinth more than once. Not only did you not know what to do with it, but it also forced you to handle elements that were completely foreign to you. Such an experience is nothing more than a consequence of breaking the interface segregation principle, which states that clients should not be forced to use methods they do not need. How would this sound in practice? Imagine that instead of one giant menu in a restaurant, you have several smaller menus corresponding to different themes, whether it's lunch, dinner, vegetarian dishes, or desserts. When you're hungry for something specific, you don't have to sift through the entire menu to find it. Easier, right?
Now let's define this principle a bit more precisely. The interface segregation principle, also known as ISP (Interface Segregation Principle), simply states that interfaces should be constructed in such a way that they do not force the classes implementing them to use methods that do not make sense for them. In practice, this means it's better to define multiple interfaces dedicated to different functionalities rather than creating one general interface that encompasses all methods. Think of it like a whole set of tools; instead of one universal tool that has a great chance of doing nothing properly, it’s better to have separate tools that perfectly fulfill their purpose. This is the key to effective code.
Doesn’t this seem logical? Instead of forcing users to use all the methods from a given interface, we isolate them into smaller, more accessible chunks. For example, let's imagine you have an interface Employee that contains the following methods:
- respondToTask()
- takeTask()
- vacation()
It might happen that an object implementing this interface, such as Manager, really needs all these methods, while an object Designer does not need to handle vacations at all. The consequence of this is that unnecessary methods turn into baggage, which will only burden the code structure and make it harder to maintain.
At what stage are you interested in practical examples of the interface segregation principle? Let's consider a code example that illustrates this principle in action:
// Define the interface for a general worker
interface Worker {
public function work();
public function takeVacation();
}
// Define more specific interfaces
interface TaskWorker {
public function assignTask();
public function respondToTask();
}
// A manager can implement both interfaces
class Manager implements Worker, TaskWorker {
public function work() {
// Manager's work implementation
}
public function takeVacation() {
// Manager take vacation logic
}
public function assignTask() {
// Assign a task implementation
}
public function respondToTask() {
// Responding to tasks
}
}
// A designer only needs to implement task-related methods
class Designer implements TaskWorker {
public function assignTask() {
// Assign a task implementation
}
public function respondToTask() {
// Responding to tasks
}
}
In this example, the classes Manager and Designer implement different sets of interfaces that correspond to their needs. We do not force the designer to sift through a tangled menu of methods that are completely unnecessary for them.
They can focus on what is important to them, and the code becomes much clearer.
Do you see how the interface segregation principle can make the code better? It not only simplifies the architecture but also increases its readability and facilitates future changes.
This is the beauty of programming based on SOLID.
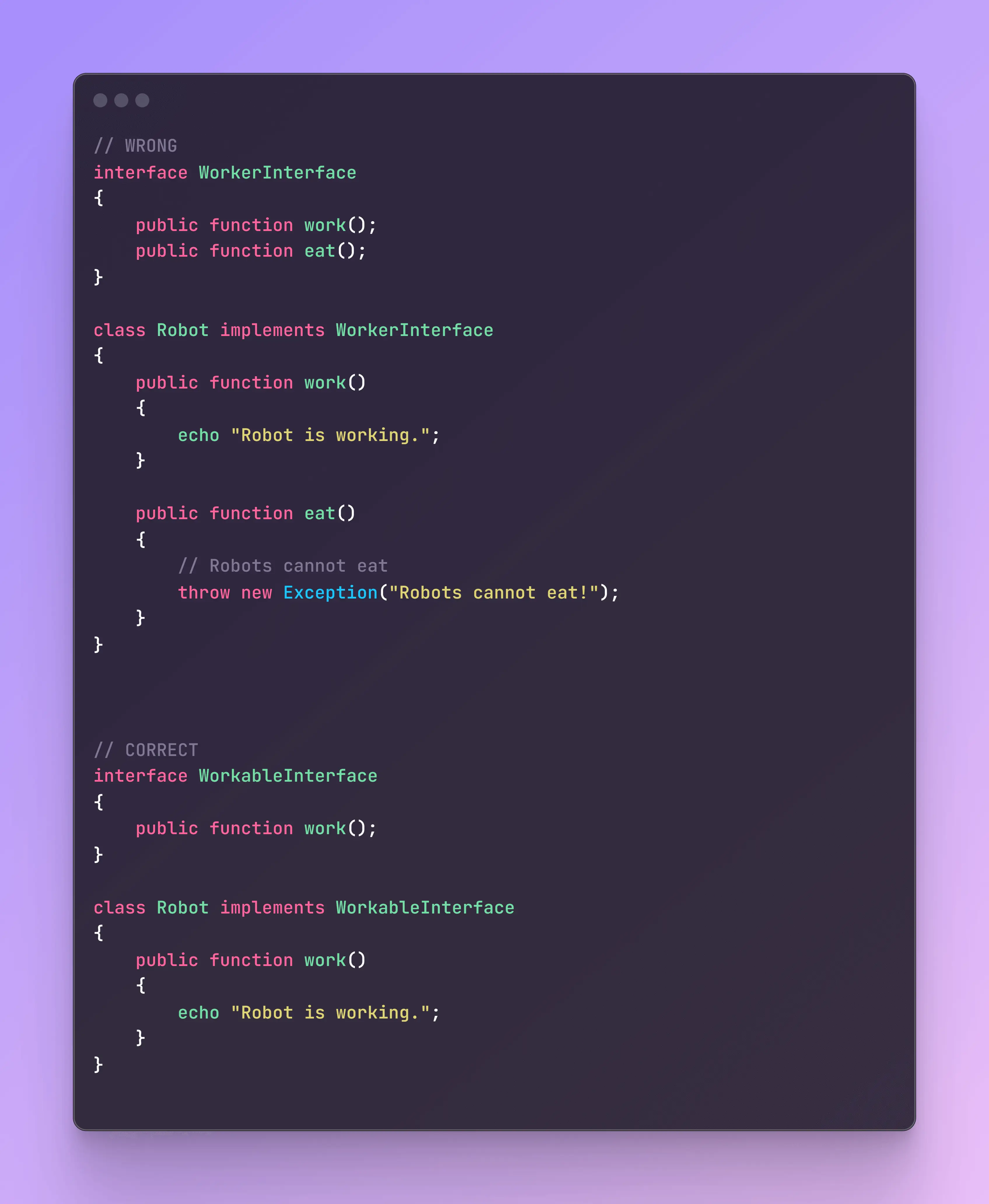
Practical Examples of Applying the 'I' Principle
When we consider the letter "I" in the SOLID principles, we are referring to Interface Segregation. Although this principle may sound a bit technical, it is actually crucial for proper application design.
Imagine interfaces as a kind of menu in a restaurant. If the menu is too extensive, customers feel overwhelmed, and their decisions can be chaotic and ineffective. Similarly, when interfaces are too cumbersome, developers face challenges in adapting their code to unnecessary methods. The aim of the interface segregation principle is to avoid this kind of mess and create smaller, more specialized interfaces that are easier to use.
Let's take a closer look at this with examples. Suppose we are creating a vehicle management application. We might start by defining an interface called Vehicle, which would include methods such as start(), stop(), fly(), and drive(). We begin with the intention of handling different types of vehicles - airplanes, cars, and bikes. But if we define everything in one interface, then for a bike, we cannot use the fly() method, and for an airplane – the drive(). How can we solve this problem?
This is where we apply the interface segregation principle. We create several smaller interfaces, such as Drivable for any vehicles that can be driven, and Flyable for flying vehicles. As a result, our interface hierarchy will look like this:
// Simple interface for driving vehicles
interface Drivable {
public function start();
public function stop();
public function drive();
}
// Simple interface for flying vehicles
interface Flyable {
public function start();
public function stop();
public function fly();
}
// Car class implementing the Drivable interface
class Car implements Drivable {
public function start() {
echo "Car started";
}
public function stop() {
echo "Car stopped";
}
public function drive() {
echo "Car is driving";
}
}
// Plane class implementing the Flyable interface
class Plane implements Flyable {
public function start() {
echo "Plane started";
}
public function stop() {
echo "Plane stopped";
}
public function fly() {
echo "Plane is flying";
}
}
In the above code, we see that the Car class implements only the Drivable interface, which means it does not have to worry about methods that are not relevant to it.
Meanwhile, the Plane class utilizes the Flyable interface. Each type of vehicle only uses the methods that are appropriate for it, which certainly makes application development easier and helps maintain clean code. Isn't that a wonderful approach?
Managing dependencies between different classes and interfaces becomes much easier. We gain significant benefits such as:
- Easier expansion to include new types of vehicles.
- High flexibility of the application.
- The ability to add new features without creating a mess.
What works against interface segregation? Excessive interfaces. While breaking down into smaller interfaces is a great idea, we must remember not to go overboard with the number of interfaces.
With an excessive number of interfaces, we might find ourselves in a situation where all these small pieces turn into an unreadable chaos. Let’s remember to find a golden mean between complexity and simplicity.
Practical application of the interface segregation principle brings real benefits in the context of application development. It not only makes our code clearer and more organized but also impacts the development team - they can work on different aspects of the application without disrupting others’ work.
This approach fosters collaboration and efficiency. Thus, the interface segregation principle is truly the king of utilizing the power of well-designed interfaces in modern programming.
After all, every good programmer knows that the key to success is the ability to manage complexity, and the interface segregation principle provides effective tools to achieve this goal. So it’s worth investing time in understanding and implementing this principle.
Adhering to the Interface Segregation Principle (ISP) brings about a number of impressive benefits that can revolutionize the way we approach system design. Imagine that you are creating a complex machine.
If all mechanisms were combined into one complex element, it would not only be difficult to operate, but repairs could also become a nightmare. The Interface Segregation Principle works on a similar principle; dividing interfaces into smaller, more specialized pieces makes our application more flexible, which is crucial in the dynamically changing world of technology.
One of the first and most important advantages noticed by developers implementing ISP is a definite increase in flexibility of their applications. When interfaces are small and specialized, it’s easier to adapt them to changing business requirements. Changes in one area of the application can be made without affecting other aspects of the system. It’s like having a toolbox — instead of one multifunctional tool that awkwardly tries to meet all your needs, you have a series of dedicated tools for each task, significantly simplifying your work.
Moreover, interface segregation leads to much easier application maintenance. When interfaces are divided into smaller fragments, code changes can be made more locally, resulting in fewer potential bugs in other parts of the application. This can be compared to repairing a car: if you want to replace the battery, it’s much easier to do this when you have direct access to it, rather than having to dismantle the entire engine compartment. This allows developers to implement fixes and updates faster and more safely, which in turn results in lower maintenance costs and higher software quality.
Let’s not forget about the excellent support for application testability. Using the Interface Segregation Principle, it's easy to create mocks and stubs for individual interfaces, which simplifies the unit testing process. This way, we can test each interface independently, allowing for quicker identification of issues and navigation through the maze of code. Imagine you are creating a computer game, and you can test each game element, from characters to environment, in isolation — this greatly enhances the quality of the final product and allows for the detection of bugs before they reach the end user.
It’s worth emphasizing that the practical implementation of the interface segregation principle also influences communication within the development team. When designers and developers have clearly defined interfaces, it becomes easier for them to cooperate and understand their tasks. It’s like in a sports team: everyone has their role to play, and when everyone knows what to do, the entire team gains efficiency.
- The ability to implement quick changes
- Simplified maintenance
- Better testability
These benefits are enough to encourage developers to take a cautious approach to creating interfaces. By adhering to the Interface Segregation Principle, we become more flexible and ready for changing market conditions. Isn’t it a visionary approach to software creation that will accelerate our development and increase customer satisfaction?
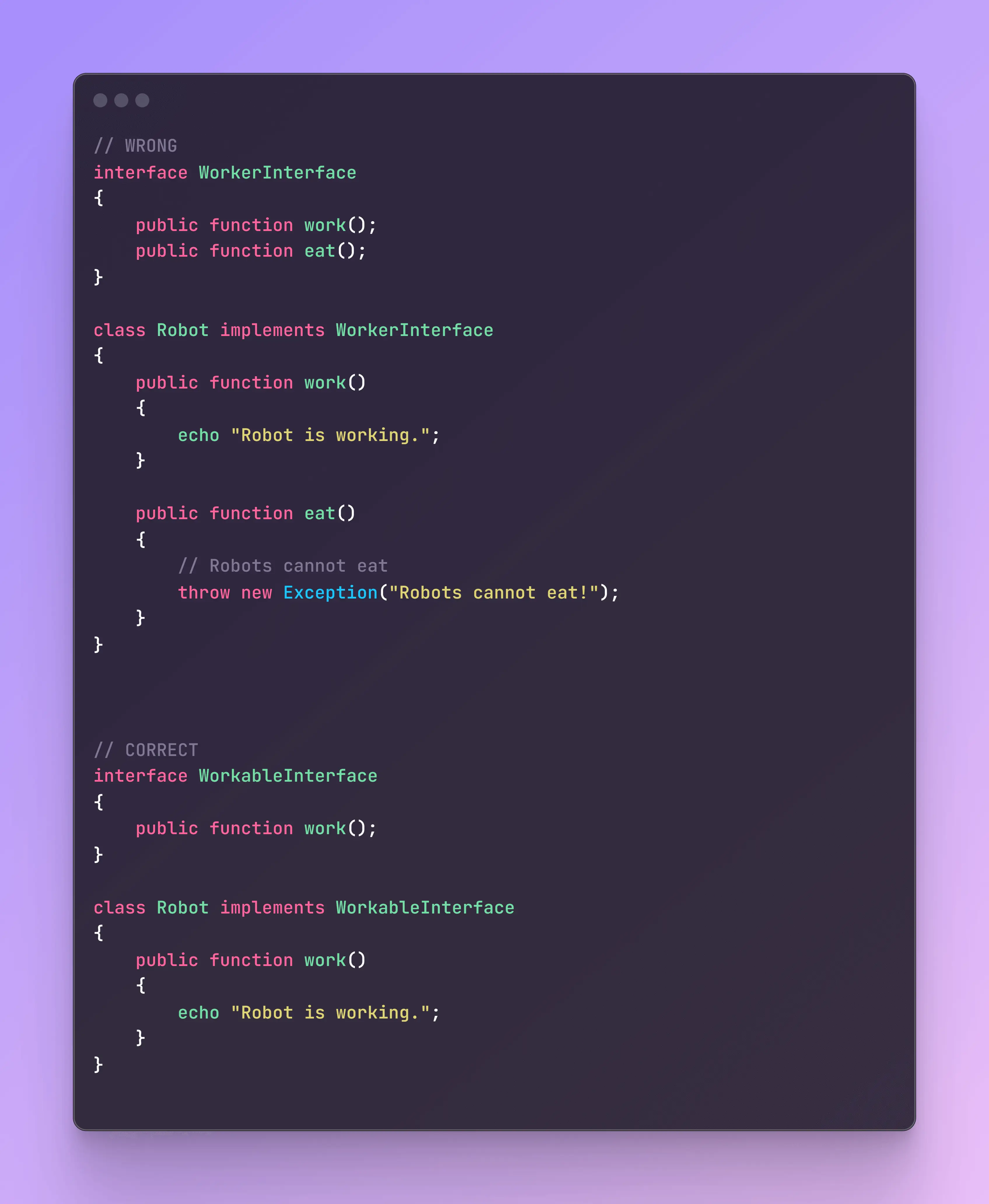
Common mistakes related to violating the 'I' principle
You know how it is: you write code intending to be elegant, clear, and easy to extend, and then suddenly everything starts to fall apart like a house of cards. Often, this is due to the improper application of the Interface Segregation Principle, the letter 'I' in SOLID. Instead of our architecture being like a well-oiled machine, we start dragging heavy burdens of complexity. But what actually goes wrong? Let’s take a look at the most common mistakes that can occur when we overlook this principle.
One of the most common mistakes is collecting too many functionalities in one interface, leading to a phenomenon known in the industry as “fat interface.”
Imagine trying to wrap all ice cream flavors into one scoop: not only will you not fit them all in, but it will probably fall to the ground too.
Similarly, with code – interfaces should be small, flexible, and specialized for specific tasks. When we start cramming them with too many methods, they become difficult to use, and cooperating with them resembles driving on a bumpy road.
Another mistake that we often forget about is not distinguishing interfaces for different clients.
If you have one interface serving multiple types of clients, you will soon have to introduce more terrible changes to the existing code, just to meet a specific client’s requirements.
It’s like trying to wear one suit for all occasions – it never looks too good. Therefore, instead, let’s ensure that each client group has its own specialized interface that meets its needs.
It’s also impossible to overlook the fact that forgetting about the responsiveness and extensibility of the code can lead to unpleasant surprises.
If you build an interface only with current needs in mind, you might find yourself in a situation where adding new features becomes unachievable.
It’s like shopping at a too small grocery store, which after a while no longer has enough room for new products. Whenever your interfaces are not segregated by responsibility, they become difficult to manage and develop in the future.
Another issue is mindlessly applying interfaces in inappropriate places.
Sometimes engineers create interfaces where it would be easier to use a class, which leads to unnecessary complication of the code.
When every piece of code requires defining an interface, we will soon open a cupboard full of dust and disorder. Ultimately, instead of simplifying life, we introduce additional chaos.
As you can see, a faulty approach to the Interface Segregation Principle can lead to mess and inefficiency in code.
Instead of focusing only on functionality, it’s worth investing time in the right structure to avoid these common traps.
Understanding how and when to apply this principle can be a key element in creating agile and flexible applications that stand the test of time.
Knowledge is a powerful tool that can transform the way we create software. Understanding the letter 'I' in the acronym SOLID is crucial for any programmer striving to create code that is not only functional,
but above all easy to maintain and develop. As we already know, Interface Segregation is a principle that states that clients should not be forced to depend on interfaces they do not use.
It's like inviting guests to a party, but not everyone wants to play on the inflatable slide. Why should everyone participate in every attraction if some of them are not interested?
The introduction to this principle, which we presented in previous sections, shows us that the key to success is to ensure that our classes and interfaces are as precise as possible.
This can be compared to a seamstress who, instead of trying to sew all kinds of clothing, decides to specialize in making dresses.
This choice not only improves the quality of her work but also makes her clients more satisfied.
We already know how important it is to apply the segregation principle, but what are the key takeaways we can draw to implement it in our daily programming? After analyzing numerous projects and implementations, here are some thoughts that may be useful for all of us.
First: create small, specialized interfaces. Instead of creating one interface that does everything, consider splitting it into smaller, more specific parts. A possible approach could look like this:
- One class is solely responsible for saving data,
- Another - for reading,
- And yet another - for validation.
This approach significantly enhances the clarity of the code.
Additionally, it's worth looking at the hierarchy of our classes. If you are creating classes that inherit from each other, ensure that each base class has a clearly defined purpose and responsibility.
Don’t forget that each class should represent only one responsibility. It’s like keeping a common dog in a cage: each breed has its own needs and preferences.
Trying to satisfy them all leads to frustration for both the dog and the owner.
Also, in the context of modern applications, note that implemented SOLID principles should be treated as a living document. Technology changes, and our approach to code does as well. It’s important to periodically revisit interfaces and adapt them to the evolving needs of the project.
Otherwise, you risk your application becoming like a cluttered attic, where you don’t know what is where.
In summary, adhering to the 'I' principle in SOLID is not just a theory, but a practical tool that impacts the quality of a programmer's daily work. Understanding how small changes in code architecture can yield huge benefits in the future,
and the ability to adapt interfaces to current needs is the key to success.
Let’s remind ourselves once again why the Interface Segregation principle is so difficult to implement: it requires not only a deep understanding of the code itself but also a vision for its future.
After all, as programmers, we should look far ahead, just as a good shoemaker plans every stitch even before the needle touches the fabric.
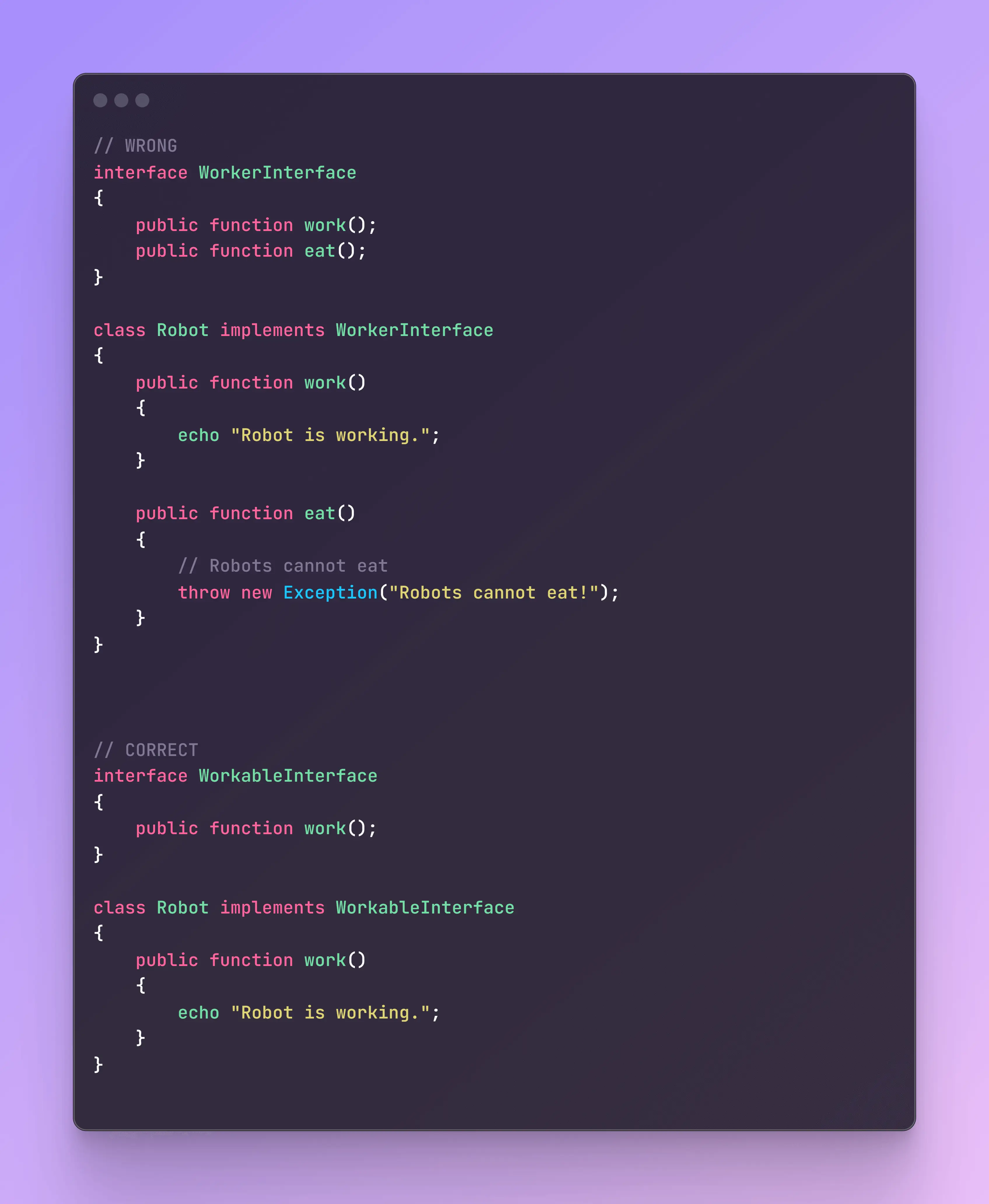