php
Introduction to SOLID
Have you ever wondered how to ensure that your code is not only functional but also easy to maintain and extend? Here comes help - SOLID principles! Now, before we dive into the details, let's try to explain what SOLID really is. You can think of it as a set of rules that serve as signposts guiding us through the winding paths of object-oriented programming. Just as traffic rules help avoid collisions, SOLID helps avoid traps that we might encounter when writing code.
The SOLID principles are an acronym consisting of five key principles that were developed by Robert C. Martin. Each of these letters represents something unique and important. Today, we'll focus on the letter S, which stands for Single Responsibility Principle. This principle states that every class should have only one reason to change, which practically means that it should deal with only one task. Sounds simple, right? However, as with many things in programming, the devil is in the details.
Imagine that you have a class in your project that handles both business logic and manages user data in the database. When the time comes to change anything in that logic, you feel terrified. What if a change in the business logic causes an error in the database operations? It's chaos, right? The Single Responsibility Principle aims to simplify such situations by minimizing the risk associated with making changes to the code.
The key to understanding this principle is also that it facilitates testing. When each class deals with only one task, it is easier to test, and any errors are easier to identify. Imagine trying to find a bug in a complicated class that does everything – it’s like searching for a needle in a haystack! Now, when the class has only one purpose, searching becomes simpler and more intuitive.
And what about practical examples? Well, we will see how the Single Responsibility Principle looks in reality. Get ready for a little journey into the world of practical applications of the S in SOLID, where examples will be within reach. In the next paragraph, we will analyze how this principle affects code structuring and what benefits come from applying it.
In the previous part, we looked at what SOLID really is and what significance this concept has in the world of programming. Today, we will focus on the first letter of this acronym, the Single Responsibility Principle (SRP). As it could be described, SRP is like a guideline that protects us from becoming a "random programmer," in a "do-it-yourself, a bit of everything" style.
What does it mean? Well, this principle states that every class should be responsible for one specific functionality or issue. Imagine a class as a restaurant – if you serve pizza, sushi, and burgers all at once, eventually you will receive criticism because everything will taste mediocre. But if you focused only on pizzas, you would surely gain a reputation as the best pizzeria in town! Benefits of this principle:
- Reduced risk of errors
- Easier testing
- Simpler implementation of changes
Speaking of the Single Responsibility Principle, it is worth introducing the concept of "reason for change." A class should have only one reason to be changed. Translating this into practice, if you need to change something in a class for different reasons, it’s time to think about splitting it into smaller components. Imagine a construction company that is involved in both building houses and designing interiors. If building regulations change, they have to change all aspects of their operations – from construction to interior decoration. How much easier it would be to do this if there were two separate companies, each specializing in its area!
In programming, this approach can be implemented by creating classes that are focused on a specific function. For example, instead of having one class named Customer that is responsible for both processing customer data and displaying it on the website, you could create two separate classes: CustomerData and CustomerView. This way, if there is a need to change the way customer data is displayed, we can do it in one place without worrying about affecting the data processing logic.
Now, let’s look at practical examples that we can use to illustrate incorrect and correct approaches to SRP. Imagine we are dealing with a class User that manages user information and simultaneously sends email notifications. This approach violates the SRP because this class has two reasons for change: changes in user data and changes in the notification system. Problems associated with this approach:
- Change in user data
- Change in the notification system
If one day we change the way emails are sent, we will have to modify this class as well as parts that do not relate to this change.
Next to this class, there should be a second one - EmailService, which will be responsible for sending emails. This way, each class will only be responsible for its task. This might seem like forced division, but in the long run, it will make our lives significantly easier. After all, it's easier to modify one small part of the code than to dig through three different places for several hours, gaining new experience.
The Single Responsibility Principle itself is the key to achieving greater readability, efficiency, and convenience in programming. The better structure we give our code, the easier it will be to maneuver through any future changes. Because as wise people say - the less chaos, the more quality. In the next part, we will analyze how this same idea can be conveniently applied in practice, and our journey through solid programming principles is just beginning!
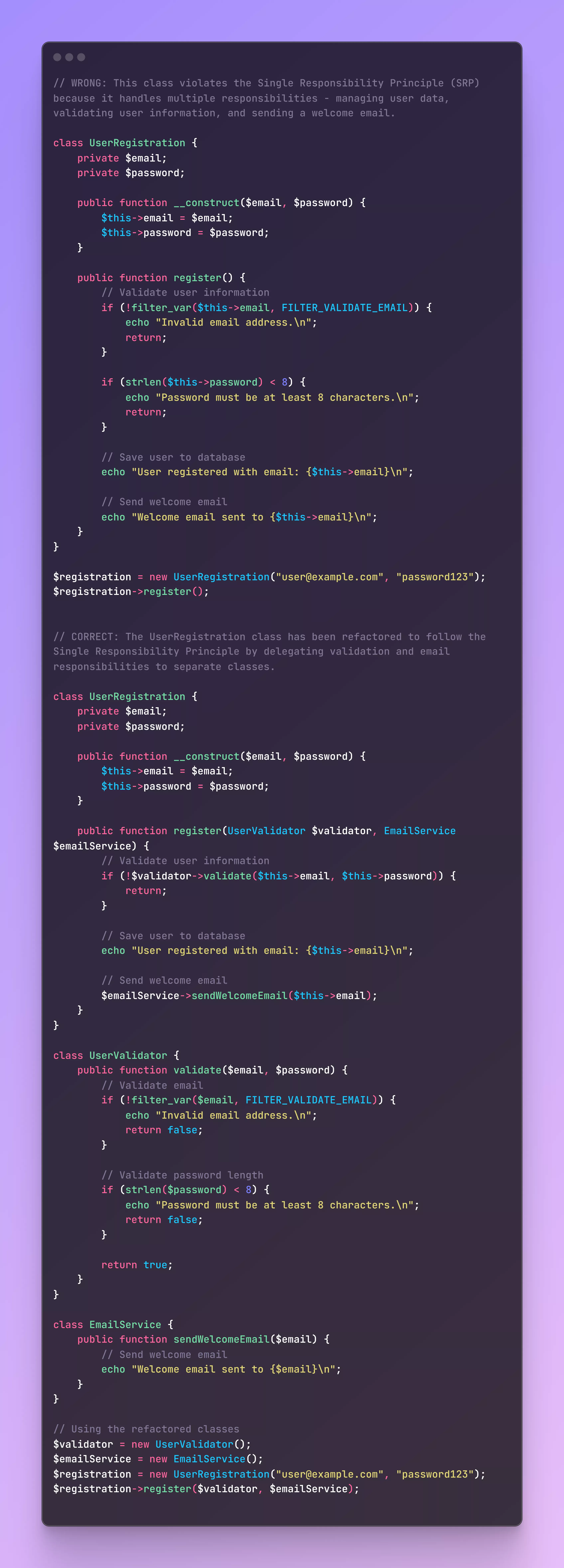
Practical Examples of Applying the Single Responsibility Principle
When we think of the Single Responsibility Principle (SRP), let's imagine an architect building a house. Each element in this building has its specific purpose and role; whether it's the windows that let in light, or the foundations that keep the entire structure above ground. In programming, just like in architecture, we can't afford to mix roles, as it leads to a chaotic and inefficient code structure. So, what does the letter "S" in SOLID actually mean in practice? Here are some real-world examples of applications that implement this principle and experience improvements in code organization and testing.
The first example could be an e-commerce application that manages customer orders. Imagine we have a class called OrderManager
that manages not only creating orders, but also payments and report generation. In the event that something goes wrong, e.g. payment processing fails, we need to understand why it specifically failed, which can be difficult if all these responsibilities are mixed in a single class. At this point, applying SRP can bring significant relief. We will separate our logic into several classes: Order
, PaymentProcessor
, and ReportGenerator
. Now each of these classes has its well-defined and clear role.
The implementation could look like this:
// Class for managing orders
class Order {
private $items;
public function __construct($items) {
$this->items = $items;
}
public function getItems() {
return $this->items;
}
}
// Class for processing payments
class PaymentProcessor {
public function processPayment(Order $order, $paymentDetails) {
// Payment processing logic
return "Payment processed for " . implode(", ", $order->getItems());
}
}
// Class for generating reports
class ReportGenerator {
public function generateReport(Order $order) {
// Reporting logic
return "Report generated for order with items: " . implode(", ", $order->getItems());
}
}
With this approach, if there is a need to modify one of the processes, for example, adding a new payment method, we can do so without affecting other aspects of the system. The PaymentProcessor
class remains clean, and we isolate changes to a specific module. How wonderful it is to have such flexibility in code?
Another interesting example could be a user management application. Imagine we have a class called UserService
, where the responsibilities include user registration, authorization, and managing their profiles. Of course, troubleshooting authorization issues can be complicated if all these functionalities are tied together in one place. Let's split this class into smaller components: UserRegistration
, UserAuthorization
, and UserProfileManager
.
// Class for user registration
class UserRegistration {
public function register($userData) {
// Registration logic
return "User registered with data: " . json_encode($userData);
}
}
// Class for user authorization
class UserAuthorization {
public function authorize($userCredentials) {
// Authorization logic
return "User authorized with credentials: " . json_encode($userCredentials);
}
}
// Class for managing user profiles
class UserProfileManager {
public function updateProfile($userId, $newData) {
// Profile update logic
return "Profile for user " . $userId . " updated.";
}
}
This division not only simplifies testing but also allows development teams to easily introduce changes. Every team member can work on a different part of the system, significantly increasing efficiency and speed of development.
In conclusion, the Single Responsibility Principle is not just a dull acronym to remember; it is a programming philosophy that brings real benefits wherever its followers aim to derive an organized and flexible codebase. In every case of application, it is clearly seen how SRP improves not only the quality of code but also the enjoyment of using and developing it. It seems that the letter "S" in SOLID is a true rock star in our programming world!
Welcome back to our journey through the land of SOLID! We continue, and today we will focus on the benefits associated with adhering to the 'S' principle, the Single Responsibility Principle. If you've been convinced by now how valuable these principles can be in the coding process, then come, sit back comfortably, and let’s discover together what lies behind this magical "S". It can be compared to cleaning your desk – either way, here you gain space that helps you be more organized.
The Single Responsibility Principle, also known as Single Responsibility Principle, is truly a major player in the world of programming, and its benefits are numerous and varied. Imagine you are in the kitchen trying to make dinner while simultaneously being responsible for laundry.:
- You can manage both tasks, but you will likely do so without much order.
- The Single Responsibility Principle works similarly in code – each class or module is responsible for one well-defined task.
This makes our code much easier to manage and understand.
An extremely useful effect of adhering to the Single Responsibility Principle is easier maintenance. When our code is split into small, independent pieces, it’s easy to understand its structure. If you find a bug in one of the modules, you won’t have to scroll through hundreds of lines of code trying to figure out what your solution was initially doing.
As we know, programmers like to enjoy their favorite piece of cake, not have a mess on their hands!
Furthermore, implementing the Single Responsibility Principle improves code readability. Anyone who has dealt with a chunk of code knows that the more complicated it is, the harder it is to understand.
- Breaking tasks down into appropriate units makes every piece of code understandable at first glance.
- This is invaluable, especially when several people are working on the same project.
- One could even say that the Single Responsibility Principle acts like a light in a dark tunnel - illuminating the way and making it easier to navigate through the puzzling maze of code.
Moreover, as the code becomes more understandable, the risk of errors decreases. The more tasks the same class fulfills, the greater the likelihood that something will go wrong.
- It may happen that changing one element affects other areas, leading to unforeseen issues.
- Separating code in accordance with the Single Responsibility Principle minimizes the risk of such errors.
- Each piece of code can be tested and improved independently of the rest of the application.
It’s like putting together a puzzle, where each piece fits perfectly without forcing the others!
All these benefits combine into one powerful force that has a transformative effect on the quality of your code.
Not only will they make your life easier, but they will also increase the value of your project in the eyes of other developers and managers.
This can be really important, especially when you are working in a team or planning to share your work with a wider audience.
So next time you feel tempted to create an "everything-in-one" class, think about all the benefits that come from adhering to the Single Responsibility Principle.
Remember, this is just one piece of our SOLID mosaic. The 'S' principle shows how powerful simplicity and readability can be in code, while what comes next will help us further strengthen our programming skills.
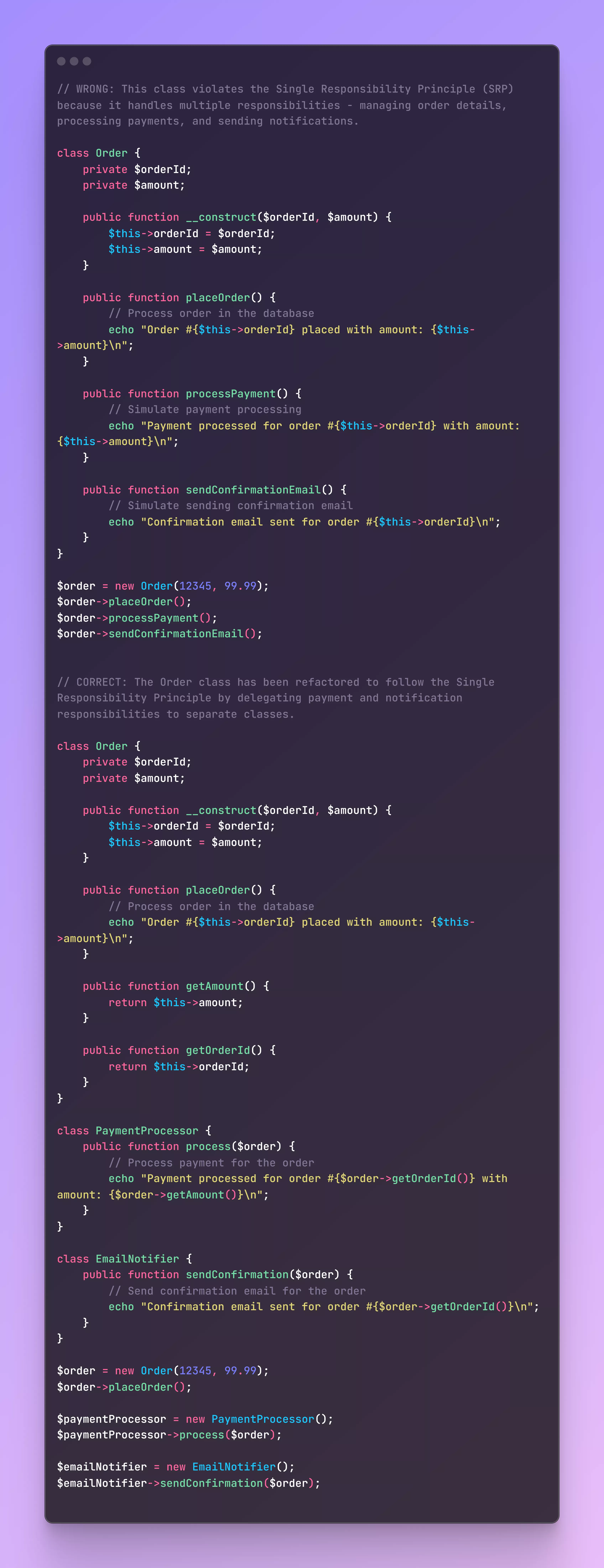
php
Key Points of Single Responsibility Principle";
echo "
$point
";
}
summarizeSRP();
?>
In light of what we have covered, one could think that the single responsibility principle is nothing more than a simple rule - each class should have its obligations within its competencies. So, if you want your classes to be as clean as returning home after a long day, you need to apply SRP like your favorite tea after hard work.
Classes that handle more than one task resemble a kitchen where spaghetti is cooked, bread is baked, and steaks are grilled simultaneously - the end result is often chaotic, and cleaning up afterwards can be a miracle.
When implementing the single responsibility principle, here are some practical tips:
- Start with small steps. Don’t try to reform the entire codebase in one night - it’s like a journey into the unknown without a map.
- Separate classes. Aim for each to become a renowned specialist in its field.
- Isolate logic. For example, you can isolate user logic in one class and the logic for database communication in another.
Another great practice is continuous refactoring. Imagine your software as a plant - it requires nurturing. Sometimes changes in one area may cause other areas to need adjustment. It’s good to regularly comb through your code, checking if classes still adhere to the single responsibility principle. If you notice that one of them starts taking on too many responsibilities, that’s a signal to act.
Don’t forget about unit tests either. Applying SRP makes testing much simpler because you deal with specific, well-defined functions. With simple classes, testing becomes as enjoyable as running on the beach - real and without unnecessary stress. After all, when you ensure that each class has only one role to fulfill, your tests will be short and concise.
Finally, it’s worth remembering that adhering to the single responsibility principle is not the end of the road. It is a philosophy that needs to be implemented in the long run, not just once while coding. Writing clean code requires staying up to date with new trends and technologies, where SRP acts as a compass in the thicket of complex code and projects. Remember, it's not just about principles, but about programming culture – that’s what true success in long-term software development is based on.
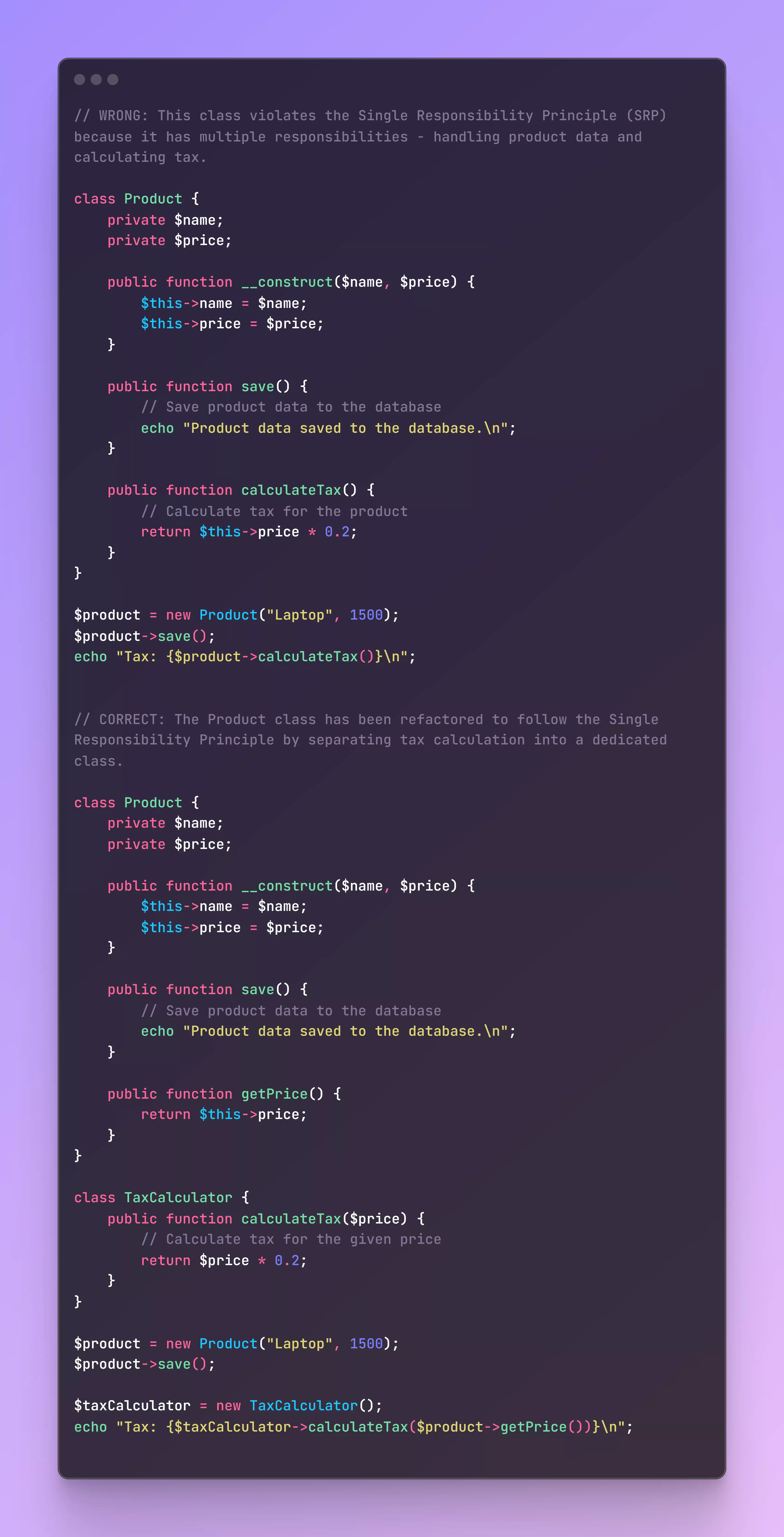