When you think about object-oriented programming, you may feel like a pilot of an airplane circling over a sea of data and code. How do you navigate the right course from this vast collection of information? This is where the SOLID principle comes in, like a compass in challenging conditions.
For your application to be not only functional but also easy to maintain and develop, it’s worth paying attention to five key principles that make up the acronym SOLID. Yes, it’s not a magic formula, but a solid foundation (as the name suggests) for effective programming.
Each letter in this acronym stands for a different principle, each playing a crucial role in the architecture of the code.
We will start with the letter 'S', and by the end of our journey, we will also touch on the letter 'O', which will be the subject of our discussion.
These principles are like traffic rules – ignoring them can lead to catastrophic consequences.
Imagine that instead of the right lane, you decide to take the left and no one knows who has the right of way. The same goes for programming. Without SOLID, your code can become chaotic and unpredictable, making it hard to modify or extend.
The SOLID principles include:
- Single Responsibility Principle (SRP)
- Open/Closed Principle (OCP)
- Liskov Substitution Principle (LSP)
- Interface Segregation Principle (ISP)
- Dependency Inversion Principle (DIP)
Each of them has its unique application and significance, and mastering them brings tremendous benefits in the long run.
By applying SOLID, your classes become more modular and easier to understand, which reduces the risk of errors and facilitates teamwork. It’s a bit like building with Lego bricks. The components are more compatible, and the structure itself is more durable.
By understanding the SOLID principles, especially the principle we will explore later, you will ensure that your projects are not only beautiful but also functional and reliable.
In the next part, we will dive into the details of the letter 'O' and discover what exactly the Open/Closed Principle means.
This principle, introduced by Bertrand Meyer, states that code units, such as classes, modules, or functions, should be open for extension but closed for modification. True, it sounds mysterious? We'll explain it step by step. Indeed, it means we need to design our components in such a way that they can be extended by adding new ones without requiring changes to the existing code. This approach ensures that our system remains more stable and easier to maintain.
Example: Imagine you are creating a project management application. As the project evolves, you need to add new features, such as:
- integration with various APIs
- the ability to add new types of tags
Therefore, instead of modifying existing classes, you can create new ones that inherit from the existing ones, allowing you to add separate extensions without undermining the foundations you already have. Consistency in code can also be supported by using interfaces and abstract classes that define common behaviors for your components.
How does this work in practice? Instead of modifying the code whenever a new need arises, it's better to design the code so that it can be "extended". This can be compared to shaping clay – instead of fiddling with fixing existing forms, you add new layers and shapes. You keep the functionality of the old production code while adding new features, transforming it into something more flexible.
For example, you could create a base class Shape
for different shapes, such as Circle
or Square
. When you want to implement something new, like Triangle
, you simply create a new class that inherits from Shape
, and you won’t break the functionality of the existing shapes. This means the project is much more future-proof, and if you encounter problems, you can focus on adding new forms rather than explaining why something in the previous code stopped working.
In practice, implementing this principle is crucial in object-oriented software design. A well-designed system that adheres to the Open/Closed Principle makes code management easier and reduces the risk of bugs in the future. So remember, being open to new ideas in programming does not mean you have to undermine the foundations – you just need to build something new on them. In the next part, we will look at practical applications of this principle in a programmer's daily work.
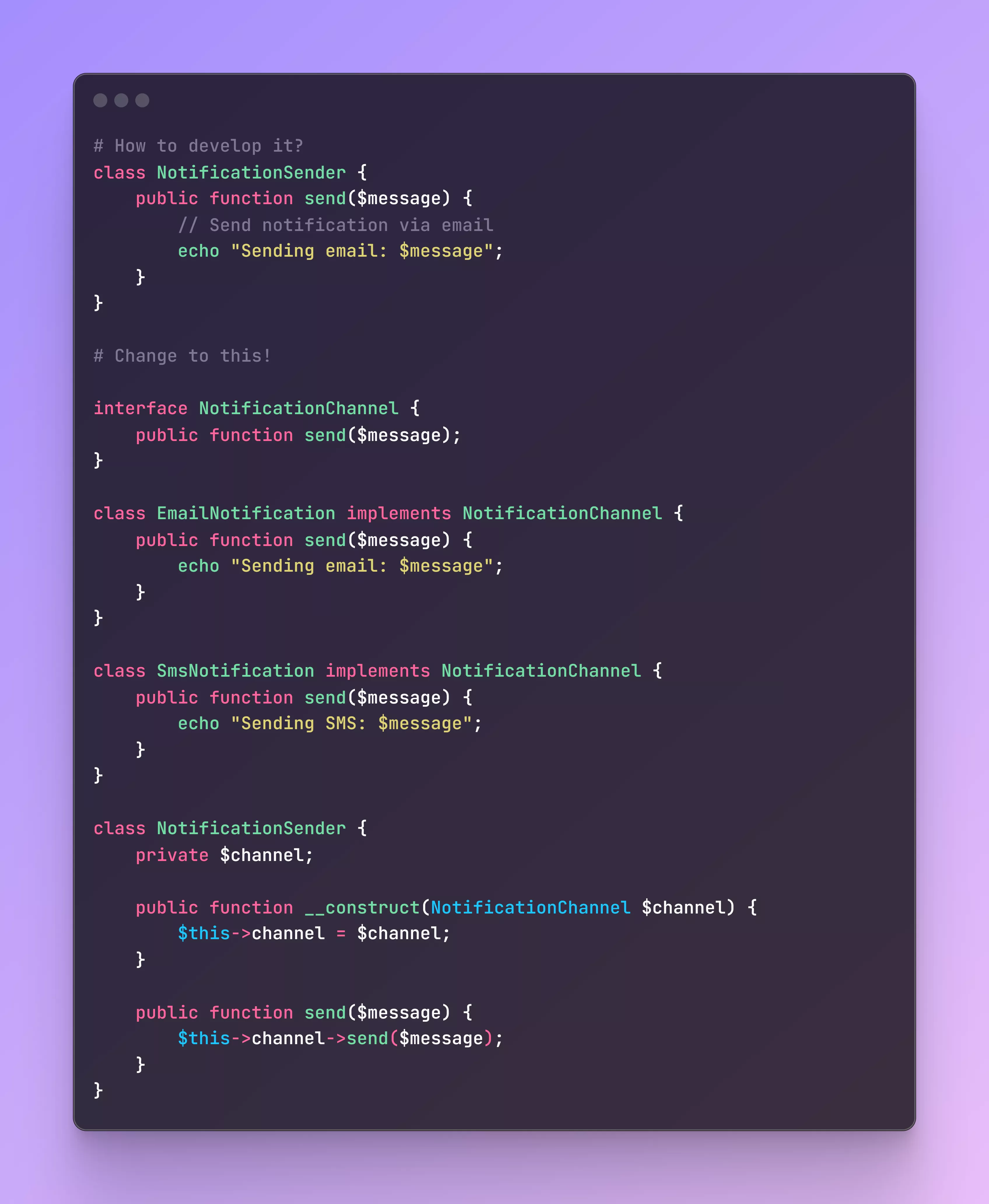
Practical Application of the O Principle
In the world of programming and software design, the O principle, which stands for Open/Closed, becomes a key element that contributes to creating more flexible and easier to maintain applications. But what exactly does this principle mean in the context of real-world projects? Imagine you are building a table. If you have to assemble every piece from scratch each time just to adapt it to changing needs, the effectiveness of your work would be, to say the least, inadequate. The O principle is nothing more than a promise that you will be able to extend the functionality of your application without the need to dig into existing code.
Now let's look at some real-world examples that show how the implementation of the O principle affects daily programming tasks.
Imagine you are creating an order management system for an e-shop. In the initial version of the program, you have only one method of handling orders. As you grow the shop and decide to add different payment methods - PayPal, credit card, bank transfer - if you do not adhere to the O principle, you start dealing with chaos. You constantly need to change the code, which only increases the risk of errors.
An example that illustrates this principle can be the strategy pattern. By implementing different payment methods as separate classes, you can create an interface PaymentMethod, and then implement each new solution in a separate class.
This way, you can add new payment methods without modifying the core code of the system. It's like adding new layers to fresh air; you can create something new without disrupting the overall structure.
Introducing the O principle impacts the scalability of the project and its simplicity. Yes, it requires a bit more work upfront, but... isn't it easier to invest time in a solid foundation than to spend time later on fixing issues? Remember that code that can be extended without modification not only saves time but also increases the program's resilience to errors. In the long run, it brings benefits like better version management and easier implementation of new features.
Let's look at one more application of the O principle that is close to every developer - reporting systems.
In the case of a classic dashboard that gathers various data, you must implement different types of reports. You can create a base report, and then for each new reporting requirement, create a tab that inherits from the same base class. This way, when you add a new report page, you only need to expand one class, and the rest remains intact.
The truth is that the O principle... can truly change the way you think about code. It works in every project, from large systems to small applications, making them more stable and less prone to failure. They have moved from ad-hoc constructions to a clear, pattern-based approach. For this reason, by investing time in its implementation, you are on the best path to creating code that will proudly stand for many years.
Let’s take a lesson from this: the O principle, although it may seem theoretical, has enormous significance in practice. Applying it in projects translates to ease of future changes and to the satisfaction of engineers who will happily return to code that did not break with every change. Why would it be any different?
Benefits of Adhering to the Open/Closed Principle
One of the key elements to consider in the context of the 'O' in SOLID is its positive impact on software development. If you imagine the programming ecosystem as a large orchestra, the O principle, or Open/Closed Principle, acts as the conductor, ensuring that everything plays harmoniously. Well, as the saying goes, nothing is perfect, but following this principle really brings us closer to the ideal!
First and foremost, the O principle encourages designing systems that are open for extensions but closed for modifications. Imagine a situation where we introduce a new feature into existing software that is already functioning well. If we had to change the original code for every new feature, we could introduce unintended bugs and destabilize the system. However, thanks to the application of the O principle, you can add new functionalities in a way that does not affect the existing elements of the system.
Moreover, you also gain better organization of the code. Treating extensions as separate modules allows you to see the daily life of a programmer not as a mosaic of chaotic elements but as a carefully thought-out puzzle. It’s like assembling jigsaw puzzles – each piece finds its place and together they create a coherent whole. Well-organized code is definitely much easier to maintain. Changes become more predictable, and every added functionality is like adding a new piece to the puzzle without disturbing anything.
In addition to order and flexibility, we cannot forget about reducing the risk of errors. When traveling through unknown roads, it is better to have a GPS than to rely solely on intuition, right? The O principle provides similar comfort in programming. When you create new features as extensions, you avoid impacting the functioning of the base code. Thus, even if you make a mistake in a new module, the risk of affecting the rest of the application is significantly minimized. Furthermore, by creating code in a way that takes the O principles into account, you can utilize design patterns that are proven and safe. This is why in the world of programming, the O principle is considered a holy grail – reconciling innovation with safety.
We also cannot forget about facilitating collaboration in programming teams. When every team member knows how to make changes without affecting existing elements, collaborative software development becomes a pleasure. In short, the O principle makes programming cease to be an eternal struggle with chaos. It creates a space where everyone can contribute by adding new possibilities to the code, like an artist adding new colors to a canvas.
To summarize, adhering to the O principle in the software development process brings numerous benefits. Offering ease in adding new functionalities, better code organization, and reduced risk of errors, this principle can become a true secret weapon for any programmer. Incorporating it into daily coding practices can transform the way we develop applications forever. Remember, good programmers are those who can not only write code but also think about its future, and the O principle is the key to understanding that.
// Example of Open/Closed Principle in PHP
abstract class Shape {
abstract public function area();
}
class Circle extends Shape {
private $radius;
public function __construct($radius) {
$this->radius = $radius;
}
public function area() {
return pi() * ($this->radius ** 2);
}
}
class Rectangle extends Shape {
private $width;
private $height;
public function __construct($width, $height) {
$this->width = $width;
$this->height = $height;
}
public function area() {
return $this->width * $this->height;
}
}
// Usage
$shapes = [new Circle(5), new Rectangle(4, 6)];
foreach ($shapes as $shape) {
echo 'Area: ' . $shape->area() . PHP_EOL; // Each shape area can be calculated without modifying the Shape interface
}
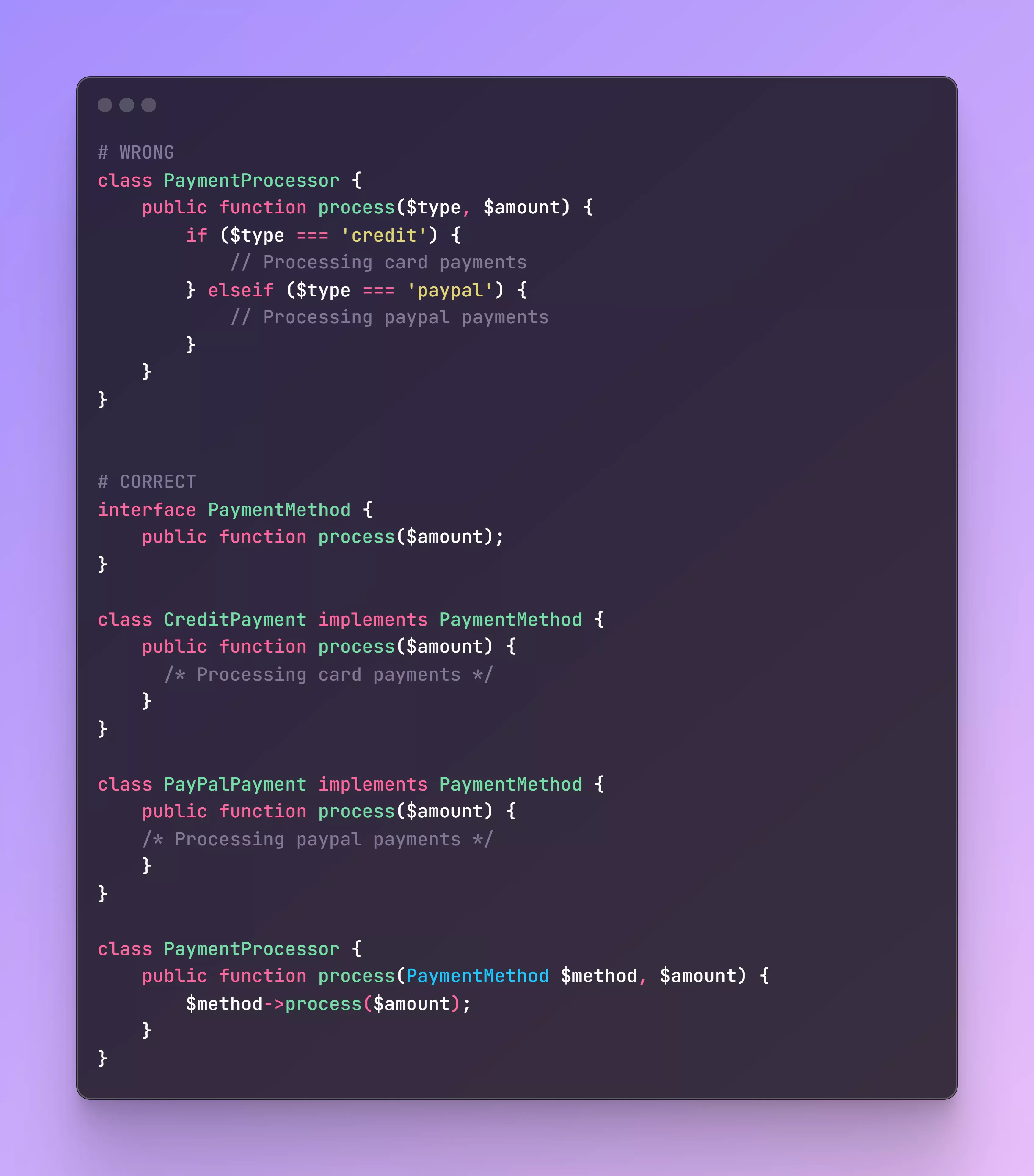
Do you know, have you ever wondered what that letter "O" in SOLID really means? If you've made it to this part of the article, you are certainly quite familiar with the principles of object-oriented programming, especially the Single Responsibility Principle. Simply put, "O" reminds us that our classes should be like versatile legs in a well-organized kitchen – each one should have its own task to ensure everything runs smoothly. When one class becomes responsible for too much, it starts to resemble a broken coffee machine that does a job but only partially, and additionally messes up everything around it.
The single responsibility principle not only makes life easier for programmers, but also makes the code more readable. After all, isn't it more pleasant to browse code that can be understood at a glance, instead of wrestling with a tangle of methods and functions that do everything at once? It's the patriot of programming – it prevents chaotic situations, just like keeping order in a closet!
In everyday practice, this principle encourages the division of different functions in a program into smaller, more manageable units, which brings several benefits:
- Ease of testing: when something is wrong, it is clear where to look for the error.
- Enhancing reusability: who wouldn't want to have code snippets that can be reused instead of writing everything from scratch?
- Ease of expansion and changes: the smaller the parts, the easier they are to modify without breaking the whole.
Surely, we all agree that sometimes true nightmares confront us – codes written according to the principle of lack of responsibility, which threaten to overturn our wonderful world of programming. And after all, no one wants to spend hours looking for a single bug in the code that might disappear if cool principles like this one were applied. For this reason, let’s remember the "O", that each piece of code should serve one purpose, making our work much easier and more enjoyable.
In summary, in programming, the single responsibility principle is a pillar of good coding – it reminds us not to get involved in unnecessary whirlwinds, but rather to explore a sensible unity of tasks. After all, ultimately, creating software is like building with LEGO bricks – the better you think through each element and its function, the more extraordinary constructions you will be able to create. A proper approach to this principle brings benefits that are evident immediately, not like in a marathon, where you have to wait a long time for them. So let’s keep the power of this principle in mind in our daily programming struggles!