Hello, programmer! Imagine that your code is a beautifully tailored suit. The SOLID principles are like guidelines for its tailoring – if you apply them well, then every clasp and button will fit perfectly, and your software will be not only aesthetic but also functional. It's a simple but strong metaphor about the importance of this set of principles in object-oriented programming.
Introduction to SOLID
We know that object-oriented programming is like assembling puzzles – each piece must fit with the rest. But what if these are not just small elements, but a whole structure built from many diverse parts? This is where SOLID comes in. Recognized worldwide, these principles form the foundation on which you can build your projects. They provide extendability, readability, and above all – ease of changes, which evokes the image of a great complex machine.
Explanation of SOLID Principles
SOLID is an acronym, but not everyone knows what it stands for. It is a set of five principles that aim to help programmers create better, more understandable, and flexible systems. These principles are:
- Single Responsibility Principle (SRP)
- Open/Closed Principle (OCP)
- Liskov Substitution Principle (LSP)
- Interface Segregation Principle (ISP)
- Dependency Inversion Principle (DIP)
Let's Focus on the Letter 'L'
Particularly interesting is the letter 'L', which stands for the Liskov Substitution Principle. This is a kind of milestone in building class hierarchies that emphasizes one key idea: subclass objects should be able to replace base class objects without affecting the correct operation of the application. Sounds like a tough challenge, right? But don't let that complexity mislead you – once you understand this principle, everything will become simpler, and your projects will experience significantly less risk of errors and greater flexibility.
As I mentioned earlier, the letter 'L' in SOLID stands for Liskov Substitution Principle, which sounds like a magic spell to some developers who are familiar with adventure movies. But don't be fooled, in reality, it's a very practical principle that can truly make a programmer's life easier. When we create classes in object-oriented programming, there arises a need for derived classes to behave as expected by those who use base classes. This can be compared to a family tradition, where every family member is expected to behave in a certain way, remaining true to their roots while also contributing something of their own.
Imagine a situation where we have a base class, let's call it Shape, and two derived classes: Circle and Square. If these classes have implementations that do not meet the expectations set by class Shape, then something has gone wrong. So how does this work in practice? If we have a method that operates on the Shape class and assumes that all shapes have a getArea() method, but the Square class returns some nonsensical value instead because it did not properly implement this method, can we still say that the Square class is actually a subtype of it? The answer is no!
The Liskov Substitution Principle states that a derived class can be substituted for a base class without affecting the integrity of the program. This means that any client using the base class should be able to use the derived class in the same way, without any concern that something might break. In fact, it is around this principle that robust applications are built, which are easy to maintain and develop. Otherwise, if we had a base class Shape and introduced some Triangle class that ignores the rules and behaves in a completely different manner, our code would be like a house of cards — easy to destroy at the slightest gust of wind.
In practice, this means that every class inheriting from the base class must implement all methods and adhere to its contracts. And what does this mean for the programmer? It means that it is not enough just to inherit from a class, but one also needs to understand its exact operation and use. It is extremely important to provide behaviors that are sensible and meet expectations, which can sometimes be a challenge, especially in large projects.
However, it is also a great opportunity for learning! By analyzing how classes inherit from one another, we can better understand how to construct our projects in a way that is solid and efficient. When a class takes on responsibilities that do not fit the original intention, it is like trying to fit a square peg in a round hole. It is not likely to work, and we would only waste time, and probably patience, which does not equate to the end result we desire.
In the context of code, this is often achieved using interfaces and abstract classes that define common methods and properties. Derived classes are obligated to fulfill these principles, which consequently allows us to create a more flexible and resilient system to changes. Additionally, Diversity of implementations can bring many benefits, but only if it remains within certain rules, which beautifully aligns with the idea of maintaining healthy code.
At the end of the day, let us remember — The Liskov Substitution Principle is not only a theoretical rule, but a guiding principle in the programming world that can help us create more effective and compatible code. Any class that fails to meet the conditions of this principle poses a threat to the stability of the entire system. After all, the IT industry is not just about coding. It’s also about ensuring that our skills contribute to creating better applications that withstand the test of time.
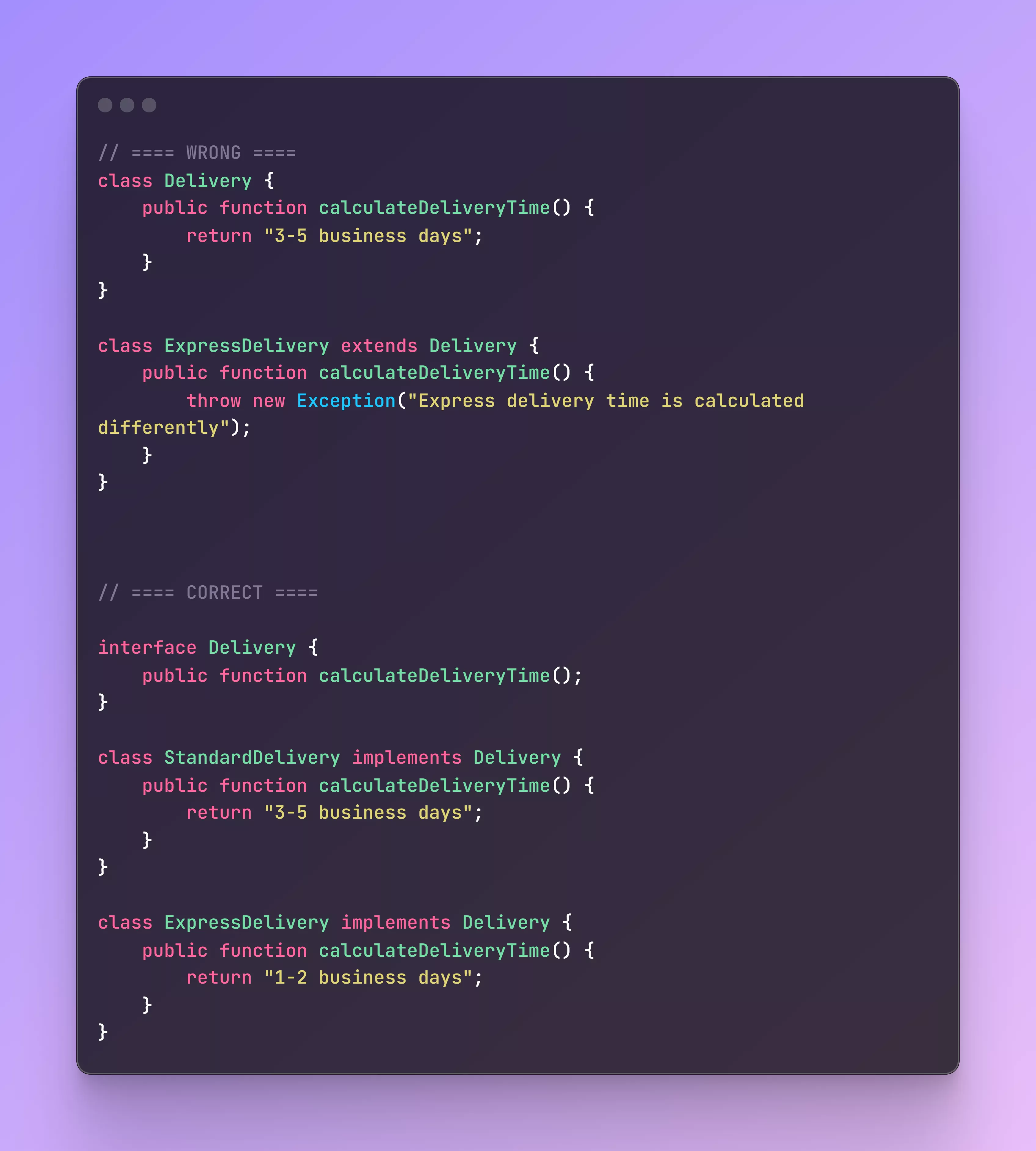
Example in Practice – L Principle in Solid
We have already established that the letter "L" in SOLID stands for the Single Responsibility Principle, and now we will focus on how this principle operates in reality. Let’s put ourselves in the shoes of a programmer who enthusiastically starts creating an application, thinking, "I wish everything was in its place."
The problem arises when we start combining various functionalities into one monolithic component. Let’s see how this can affect our code.
Imagine a class that manages both the main operations and various auxiliary functions. The team has decided to create a class UserManager
, which not only deals with user management but also sends email notifications and logs user actions. In theory, this sounds nice, but in practice, our dreams can quickly turn into a nightmare.
// Example of a class violating the Liskov substitution principle
class UserManager {
public function registerUser($userData) {
// Registration logic
$this->sendEmail($userData['email']);
$this->logAction('User registered: ' . $userData['email']);
}
public function sendEmail($email) {
// Logic to send email notification
echo "Sending email to $email";
}
public function logAction($message) {
// Logic to log user actions
echo "Log: $message";
}
}
In the example above, the class UserManager
violates the Single Responsibility Principle. Its functions are lumped together into one structure, leading to many problems. Think of it as carrying too much luggage on a trip — the more we have, the harder it is to carry. What happens if we forget some things in the luggage? It may turn out that we won’t reach our destination as quickly as we wanted, and our journey will become more stressful than enjoyable.
Here are five reasons why overloading tasks in one class can lead to disaster:
- Difficulties in testing: When a class is responsible for many functions, testing each of them becomes a challenge. We have to test each function in the context of others, which introduces variability and potential confusion.
- High complexity: Too many responsibilities in one place lead to complexity. When a class requires understanding of literally all aspects of its operation, it is difficult to predict how changes in one function may affect the rest.
- Difficulties in development: Imagine we want to add a new notification feature, such as SMS. We need to start restructuring our class, which can lead to larger problems if not done properly.
- Unreadable code: A long class with many methods becomes unreadable. Other developers taking over the project will find it much harder to understand what is actually happening in this "one big piece of code".
- The concept of responsibility: Over time, our priority should be clarity of responsibility. When a class exceeds its small space, using it becomes annoying and inefficient.
The above problems clearly illustrate the negative consequences of violating the Liskov Principle. We can assume that introducing these changes will cause our example class UserManager
to become even more complex. But how can we fix this? It’s very simple – we resort to the Single Responsibility Principle in the next step, creating cleaner and more organized code.
We can extract responsibilities into separate classes, which will help us avoid the tensions associated with testing and maintaining the code. An example of this approach looks like the following:
// UserManager class handling only user registration
class UserManager {
public function registerUser($userData) {
// Registration logic
echo "User registered: " . $userData['email'];
}
}
// EmailService class handling email notifications
class EmailService {
public function sendEmail($email) {
// Logic to send email notification
echo "Sending email to $email";
}
}
// Logger class responsible for logging actions
class Logger {
public function logAction($message) {
// Logic to log user actions
echo "Log: $message";
}
}
Now each class focuses solely on one responsibility, making the code more understandable and easier to test. The class UserManager
is only responsible for user registration, while all other tasks have been moved to their own classes. As you can see, the Liskov Principle and adherence to the Single Responsibility Principle elevate our code to a higher level and also make our collaboration with the team more efficient.
// Usage example
$userManager = new UserManager();
$emailService = new EmailService();
$logger = new Logger();
$userData = ['email' => 'test@example.com'];
$userManager->registerUser($userData);
$emailService->sendEmail($userData['email']);
$logger->logAction('User registered: ' . $userData['email']);
With this approach, our journey into the world of SOLID becomes an exciting adventure, and every change in the code is simpler and more enjoyable. We will no longer waste time solving problems related to the traps of complex code. We are already one step closer to excellent software quality.
In previous sections, we explored the basics of the Liskov Substitution Principle, and now we will return to the practical dimension of this concept. It is worth considering why this principle is so crucial in modern programming. After all, who wouldn't want their code to be not only efficient but also understandable and easy to maintain? The mouse in your pocket (I'm referring here to linear dependencies) suggests that maintaining a comfortable workflow on a project depends on how well you adhere to good programming practices.
Adhering to the Liskov Substitution Principle affects many aspects of a programmer's daily life. For example, when you have a base class and various derived classes that implement the specifications of the base class, you can gain significant benefits. Imagine that the base class is a house, and the derived classes are different rooms – each has its function, but together they create a cohesive whole. If you change something in one of the rooms, the rest of the house remains intact. This is precisely because of principle L that you can flexibly interchange and modify classes without the risk of compromising programming functionality.
The benefits of adhering to the Liskov Substitution Principle are undeniable:
- Improved code testability: When classes are interchangeable, you can easily create unit tests for each of them, making bug detection much simpler.
- Elimination of issues: Tests can be conducted at the base class level as well as at the derived class level, resulting in greater confidence in your code.
- Reduced risk of errors: If you can replace one class with another and obtain the same result, it means you have written the code in accordance with principle L.
We must not forget about code maintenance, which also gains in value. Imagine that you are dealing with many classic cars. Each of them has its specific parts – but some of them are interchangeable. When you make changes to one model, you can be sure that other models, which are based on the same construction principles, will also work without issues. When a project is built on solid foundations of adhering to principle L, maintenance becomes less time-consuming and more predictable.
Ultimately, one can say that the clarity of software architecture is a key asset of adhering to the Liskov Substitution Principle. Instead of struggling with chaotic code structures, the programmer gains a clear architecture that is logical and intuitive. Derived classes can be easily understood because of their reference to base classes, making teamwork more efficient. This is reminiscent of how we learn foreign languages – understanding grammatical rules facilitates communication and understanding.
Adhering to the Liskov Substitution Principle is nothing more than focusing on quality aspects in programming – the resulting benefits are a treasure trove of practical advantages that every programmer should have in their dictionary. After such a dose of information, it is clear how crucial this approach is in daily practice, so that our software not only works but also makes the work on it enjoyable.
![```html
<h1>Example Code Illustrating the Proper Use of the Liskov Substitution Principle</h1>
<p>The Liskov Substitution Principle (LSP) states that objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program.</p>
<pre>
<code>
class Bird {
public void fly() {
System.out.println("Flying");
}
}
class Sparrow extends Bird {
@Override
public void fly() {
System.out.println("Sparrow is flying");
}
}
class Ostrich extends Bird {
@Override
public void fly() {
throw new UnsupportedOperationException("Ostriches can't fly");
}
}
public class Main {
public static void letBirdFly(Bird bird) {
bird.fly();
}
public static void main(String[] args) {
Bird sparrow = new Sparrow();
letBirdFly(sparrow); // Works fine
Bird ostrich = new Ostrich();
letBirdFly(ostrich); // Throws an exception
}
}
</code>
</pre>
<p>In this example, the <strong>Bird</strong> class is a superclass, and <strong>Sparrow</strong> and <strong>Ostrich</strong> are subclasses. The <strong>letBirdFly</strong> method expects a <strong>Bird</strong> type, but when it tries to use the <strong>Ostrich</strong> instance, it fails to fulfill the LSP. In this case, the <strong>Ostrich</strong> violates the principle because it cannot simply replace the <strong>Bird</strong> type and behave correctly.</p>
<p>To correct this, we could use interfaces or redesign the class hierarchy to ensure that all subclasses can fulfill the expected behavior.</p>
```](https://oatllo.pl/storage/uploads/1731693526.webp)
Practical Tips for the Liskov Principle
As part of our journey into the mysteries of object-oriented programming, particularly regarding the Liskov Principle, we have reached key conclusions that will allow you to fully leverage the potential of this fundamental concept. Yes, the letter 'L' in 'SOLID' stands for Liskov Substitution Principle, and understanding and applying this principle can be nothing less than a golden key to success in your programming projects. So, to not keep you in suspense any longer, let's take a look at best practices that are worth implementing.
First and foremost: always remember that subclasses should be fully interchangeable with their base classes. Like a beautifully constructed house of cards – if one of the cards is incompatible, the whole structure starts to wobble. Similarly, in programming, use subtypes that can be successfully substituted for supertypes, without affecting the system's operation. Moreover, when creating new classes, make sure they inherit all relevant behaviors and attributes from the base class.
Next, maintaining the appropriate class hierarchy not only affects code flexibility, but also readability. Isn't it incredibly satisfying when the code structure resembles a well-organized library where you can find everything you need? What is essential in such a system is consistency in implementation. When a derived class changes the behavior of a base class, it should do so in a predictable manner. Otherwise, we get a domino effect, where every change leads to chaos in the code.
In the context of class design, try to avoid using implementation details of base classes in derived classes. This way, we do not create unpleasant entanglements that complicate changes. As the saying goes, “the less you know about the other side, the better”. So that we can manage changes when the need arises, write code in a way that doesn't depend on internal details.
Don't forget about testing! Testing derived classes should confirm compliance with base classes. Nothing reinforces a programmer's confidence more than the first test that confirms everything works as expected. A successful outcome is like a reward, while a failure is a sign that something needs fixing. Although it sounds like a cliché, in practice, we often forget about it!
Thus, translating the letter 'L' in SOLID into specific actions, we can state that every subclass should adhere to the principles of peaceful unease. Creating code that solves problems, rather than multiplying them, ensures that your applications will be not only functional but also aesthetic and easy to maintain.
By utilizing the above tips, your project will gain stability, and you as a programmer will feel the satisfaction of a job well done. As John Wooden said: “Success is peace of mind”, and in programming, this also applies to our little, yet impossible to overlook letters. Learn, act, and the results will come naturally, like flowers after summer rain.
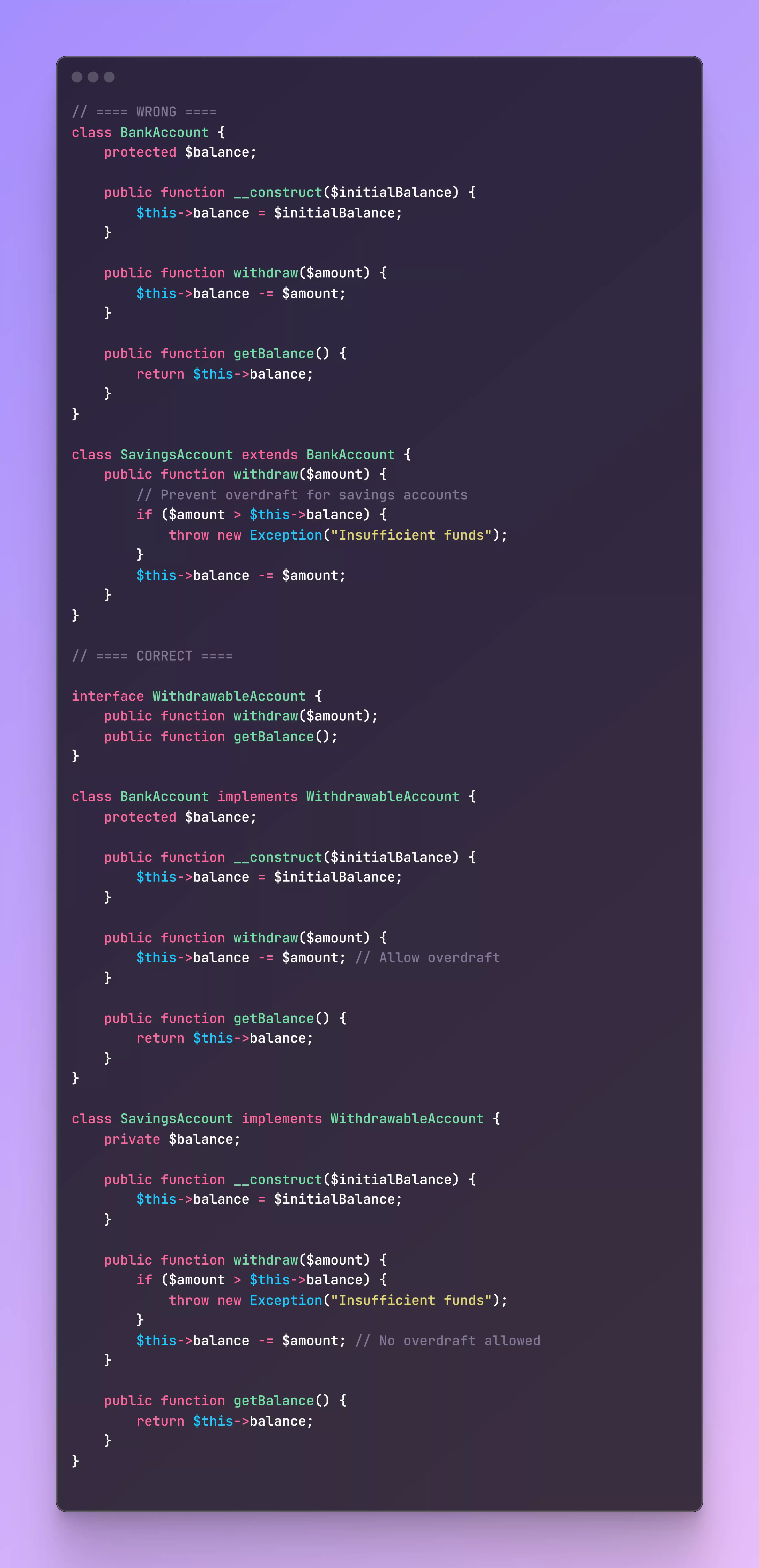