Introduction to the Dependency Inversion Principle in SOLID
In the world of modern application programming, we often face the challenge of making our code readable, flexible, and easy to test. One of the main tools that help achieve these goals is a set of principles known as SOLID. Today, we will take a closer look at a principle that is extremely important for software architecture, namely the dependency inversion principle.
Sounds complicated, right? But don’t worry, I’m not going to introduce you to the world of programming using convoluted jargon! Imagine that you are building a house; in that case, you wouldn’t want all the walls to be built using expensive materials that complicate future changes to the layout of the rooms. That’s exactly what the dependency inversion principle does – instead of myths and illusions, it changes the way we should think about relationships between our classes, as if they were the walls in our beloved home.
More formally, the dependency inversion principle states that high-level modules should not depend on low-level modules. Both should depend on abstractions. Abstractions should not depend on details, details should depend on abstractions. What does this mean in practice? It can be compared to having administrative decisions at the top that are independent of decentralized execution, thus ensuring greater flexibility.
Let’s imagine a scenario, where you are writing a book management application. In a traditional approach, the Library class might directly use the BookFrame class, so any changes in how books are stored (whether in an external database or JSON files) would require changes in the Library class as well. The dependency inversion principle prescribes that the Library should not directly depend on BookFrame, but rather on something we call an interface. This way, for example, we can introduce new ways of storing books without fear, maintaining full control over the rest of our code.
If all this is related to architecture, the dependency inversion principle acts like a support that guarantees stability. Without these supports, our code could quickly fall apart under the weight of changes and fixes. That’s why adhering to this principle when writing code is so important – it’s like having a foundation on which to build more complex structures.
So, the next time you hear about dependency inversion, you can think of an architect planning a house. Not only does he think about his constructions or materials, but also about how they all interact with each other. This explanation may seem simple, yet it is so often overlooked in everyday programming. Following the dependency inversion principle can greatly ease the lives of developers, allowing them to write more flexible and maintainable code.
You know, not every programmer has an easy life - especially when various design principles, such as the Dependency Inversion Principle, appear on the horizon. But it's okay, don’t stress! Today, we’ll dust off this stylish concept from the realm of SOLID. So let’s start from the very beginning: what exactly is hiding behind this mysterious term?
When we think about dependency inversion, imagine you have buildings that depend on foundations. In traditional programming architecture, it is the foundations (i.e., the details) that must be tailored to the buildings (i.e., the abstractions). It’s a bit like building a sandcastle – you need to have a solid foundation for the castle to stand. But ultimately, the dependency inversion principle tells us something entirely different: the foundations must be flexible, so that different buildings can stand upon them. The main point of this approach is that we cannot let our details govern the entire structure.
Now you might wonder, why should we be thrilled about this? Well, in a false belief that our classes must be tightly coupled, we often create a tangle that I think resembles spaghetti code. Instead, we want to introduce abstraction – and this is the key to understanding the dependency inversion principle. Let our modules depend on interfaces rather than concrete implementations. At this point, our code begins to have greater flexibility, leading to easier testing and modifying.
Let’s look at it from another perspective. You see how in many action movies the hero faces danger? At some point, they give up a toxic, consuming relationship with more destructive elements. And that’s exactly what we want to achieve in programming by applying the dependency inversion principle. Instead of engaging in complicated relationships, we would prefer our classes to be decoupled, with communication occurring through abstract interfaces. That’s real magic!
Returning to examples: let’s say you have a payment processing application.
In one approach, writing code that directly depends on a specific payment platform will lead to cumbersome updates every time something changes on the external provider’s site.
But with the dependency inversion principle, you create an interface that defines what actions can be performed. Then, you implement those actions in dependent classes.
This means that changes in the external entity won’t impact your wonderful, overarching code.
Ultimately, this principle not only enables us to create more complex systems but also makes our lives as programmers significantly easier.
- Exceptionally strong ties between classes? No.
- Pattern layout – base – interfaces – implementations? Yes!
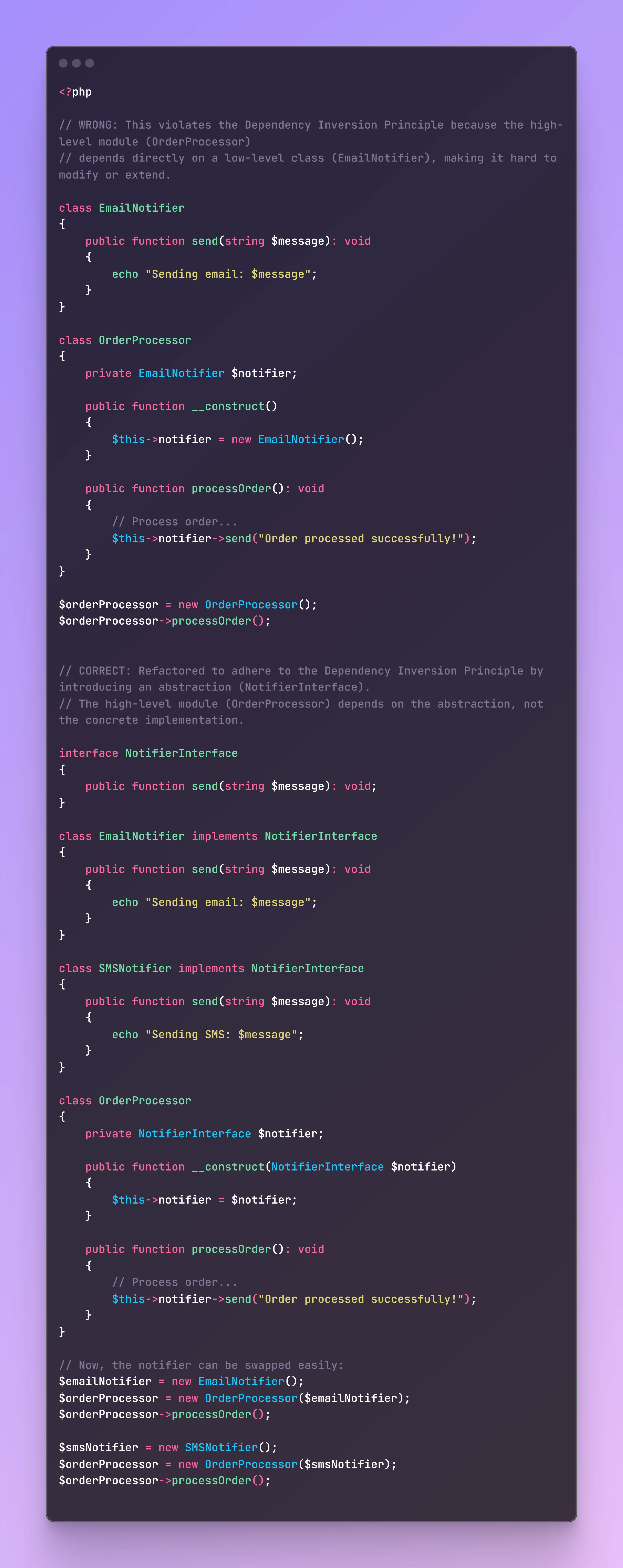
Advantages of Using the Dependency Inversion Principle
Welcome to the world of dependency inversion, where the SOLID principles are not just a theoretical concept, but a true key to success in creating software that not only works but also has longevity. Have you ever wondered why some software systems are like reliable Swiss watches, while others resemble paper airplanes—catchy but short-lived? The answer may lie in the dependency inversion principle. Today, we will explore what this principle brings to our code and how it helps in its improvement.
The first significant advantage to highlight is increased flexibility. No idea what I mean? Think of dependency inversion as a magic spell that allows your modules to freely swap external dependencies.
When we create interfaces that separate implementation details from client code, we gain greater freedom in choosing libraries and frameworks. Want to swap one implementation for another? Go right ahead, just change a few lines of code. It's a bit like changing an engine in a car without having to move the entire body. Simple and convenient!
Furthermore, reducing coupling in the system is another amazing advantage. When our components are tightly coupled, changing one of them can introduce total chaos in other parts of the system. Thanks to the dependency inversion principle, we lose this problem like an awkward ballet dancer would lose balance during a complicated performance. Instead of keeping all elements close together, we allow them to become more independent, which means that changes in one part of the system don't have to force changes in others. An ideal example is minimizing the impact of changes on databases in the context of different application layers.
What about testability? I often say that code is not just functions, but also promises. Good code should be testable at every stage of its development. This is where the dependency inversion principle steps onto the stage like a superhero. By introducing abstractions that are used in tests, we can easily simulate external dependencies. Imagine you want to test complex business logic, but you're afraid that the external service won't respond or is too slow. Thanks to dependency inversion, instead of connecting to the actual service, we can substitute our own test interface. It’s like having your own laboratory, where instead of experimenting on a real animal, you use simple models to test your hypotheses.
As our system evolves, we avoid the monolith trap where every change requires a massive amount of work and thought. This is the perfect moment to see the dependency inversion principle as a guardian of efficiency. The ability to swap individual components and maintain them in the system as modular units makes the application more resilient to external changes, as well as future expansions.
All these advantages certainly translate into better code quality and a more enjoyable programming experience. The dependency inversion principle is not just a set of rules; it is a fundamental concept that allows developers to breathe at a leisurely pace, as well as trust their work. Increased flexibility, reduced coupling, and better testability—captivating, right? Like music in our ears, relieving the headache that often accompanied us during programming.
In the long run, the dependency inversion principle transforms our code into a true symphony, and developers into classical conductors—managing their ensemble so that everything plays together. So remember, in the world of programming, flexibility is key, and the dependency inversion principle is a key tool for unlocking it.
Application of the Principle in Practical Projects
When we talk about the dependency inversion principle, we cannot forget how much it can simplify a programmer's life.
Imagine that your application is a beautiful multi-story building, and the dependency inversion principle is its solid foundation.
Without them, the entire construction might collapse, right? Today we will look at how this principle works in practice and what steps to take to actually benefit from its advantages.
To start, let's consider a project that many of us know: a task management application. Traditionally, in such an application, business logic often rigidly depends on external libraries, which can lead to lack of flexibility.
Why not try to apply the dependency inversion principle to simplify everything? How does it look in practice? Instead of creating strong ties between components, let's define interfaces that allow every element of the system to easily "connect" to different implementations.
This means that we can swap one class for another at any moment without affecting the rest of the system.
An example? Let's say we have a class TaskManager
that manages tasks. Without applying the dependency inversion principle, its code might look like this:
// Without Dependency Inversion
class TaskManager {
private $dbConnection;
public function __construct() {
$this->dbConnection = new DatabaseConnection();
}
public function addTask($task) {
// Code to add task to the database
}
}
As you can see, TaskManager
is strongly coupled with DatabaseConnection
.
Now let's introduce an interface, making our code more flexible:
// With Dependency Inversion
interface TaskRepository {
public function addTask($task);
}
class DatabaseConnection implements TaskRepository {
public function addTask($task) {
// Code to add task to the database
}
}
class TaskManager {
private $repository;
public function __construct(TaskRepository $repo) {
$this->repository = $repo;
}
public function addTask($task) {
$this->repository->addTask($task);
}
}
As you can see, now TaskManager
no longer directly depends on a specific database implementation.
It's like you swapped a universal remote you bought at the store for a dedicated one. You can switch it for another and not worry about changes in your collection of gadgets.
Moreover, consider the usefulness of design patterns such as Factory Method or Strategy Pattern, which can work great with the dependency inversion principle.
Imagine that you are creating a system with various strategies for processing tasks - everything can be implemented while maintaining clean code and application flexibility.
When designing systems, remember that the dependency inversion principle not only increases the testability of your code but also makes it more understandable for other programmers.
With such code organization, one could say that it even invites the next developers to the project.
It resembles an organized party where everyone knows what they are doing and how they work with other guests.
As the dream of simplicity and flexibility becomes a reality, don’t forget about documentation.
The dependency inversion principle, while simple in its assumptions, requires clear understanding from all parties involved.
Remember, a good project guide is the best way to help others understand how and why this principle was applied.
Just like building a bridge, you need clear plans that help travelers cross to the other side, regardless of how complicated the conditions on the road may be.
Examples of applying the dependency inversion principle in practice are as diverse as LEGO bricks - by creating a cohesive structure, you can build almost anything!
Are you ready to pick bricks for your new application? Let the principle of inversion be your best guide through the chaotic world of coding.
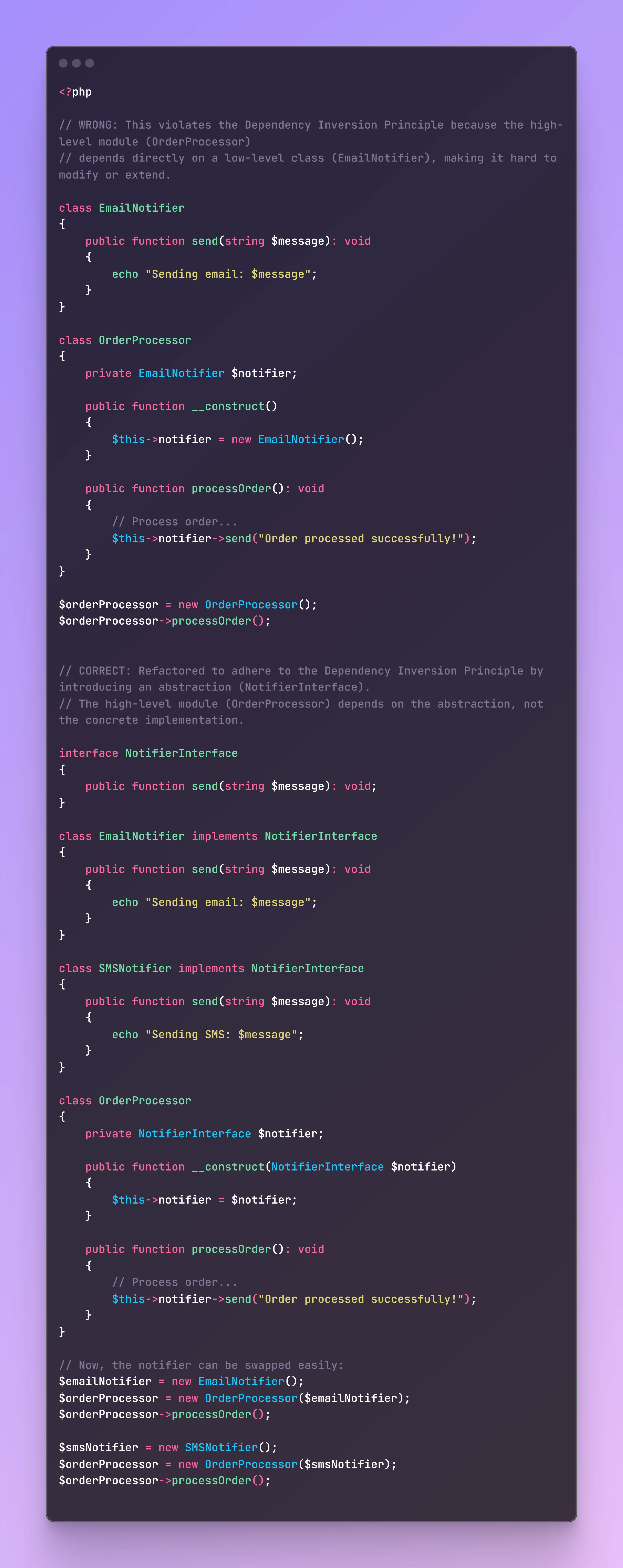
Although the principle of dependency inversion, which is one of the cornerstones of SOLID principles, is an excellent tool in a programmer's arsenal, it is easy to fall into traps that can harm the entire project. As the classic said, "learning is not a simple matter", and the field of programming is rife with subtleties that can trip up even the best specialists. Let's take a look at the most common mistakes and traps associated with implementing this principle, which can lead to not only frustration but also complicated architecture and difficulties in the later maintenance of the code.
Most common traps related to dependency inversion
- Trap 1: Introducing interfaces too early
Some programmers, in pursuit of implementing the dependency inversion principle, may introduce interfaces at the very beginning of the project, even before determining what will actually be needed. Imagine a painter who orders canvas and paints before even knowing what they want to paint. Of course, the good intention is a desire for flexibility, but such a step leads to confusion and excessive complexity in the code. In practice, it is much better to let the project evolve, introducing interfaces as needed, which helps maintain simplicity and transparency in the application's architecture.
- Trap 2: Ignoring dependencies in testing
Testing is a key element in programming, so it's no surprise that improper management of dependencies can cause serious problems. The verdict on our project may be passed as soon as we ignore the principles of dependency inversion, creating strong ties between classes. An example could be a situation where a class deliberately refers to a specific implementation instead of an interface. As a result, unit testing becomes a real nightmare because we cannot easily mock dependencies. To avoid this, it is worth adopting an approach where interfaces are clearly defined, and the classes implementing them are treated as trainers, ready to provide their skills for testing.
- Trap 3: Overloading interfaces
Interfaces, like superheroes, have their limitations. If someone places too many expectations on one interface, it will likely cease to perform its function—instead of helping, it will start working against us. Imagine a gigantic robot with hundreds of buttons, each serving different tasks. Eventually, when you encounter a problem, it won't be clear which button to press. Instead of one powerful interface, it's better to divide responsibilities into smaller, more understandable interfaces. This helps manage complexity and makes the system more modular.
- Trap 4: Inconsistent application of the principle
Different aspects of an application can be designed in different ways, leading to inconsistency throughout the system. When one piece of code is written according to the dependency inversion principle while another is not, it becomes a battlefield fraught with errors and confusion. A unique approach to applying SOLID principles throughout the project is crucial. Once again, the comparison to a concert schedule comes to mind—it would be wonderful if all musicians played in the same rhythm instead of each playing their melody in their own tempo.
- Trap 5: Basing the application on specific implementations
Creating strong dependencies between classes and specific implementations is nothing short of pushing a cart without wheels. This makes our code complicated, difficult to expand, and it gets replaced as quickly as possible because we fear being left with old, narrow solutions. When planning the structure of your application, focus on the interfaces. Everything from application logic to the database should be designed in such a way that it is not tied to anything specific, giving us great flexibility in future development and changes.
It is worth remembering that the principle of dependency inversion, while wonderful, is not a cure-all for all programming difficulties. When well understood and applied, it can significantly enhance our work and lead to agile and elegant solutions. On the other hand, making bad decisions in this area can cost our project dearly. However, with knowledge of common traps and techniques to avoid them, we can better prepare for any challenges in the programming world.
Comparison of the Dependency Inversion Principle with Other SOLID Principles
If we think of SOLID principles as the rules of a chess game, the Dependency Inversion Principle (DIP) plays the role of a crucial move that can completely change the course of the entire game. When it comes time to compare DIP with other principles, like in an exciting tournament, every move matters, and each principle is part of a well-thought-out strategy. The individual SOLID principles are the renowned five rules: single responsibility, open-closed, Liskov, interface segregation, and dependency inversion. How do they interact and what connects them? Let's begin this fascinating journey!
The single responsibility (Single Responsibility Principle, SRP) reminds us that every Module, Class, or Function should have only one responsibility. It's like building a Swiss watch—each cog should work separately but in perfect harmony. By applying SRP, you create very cohesive components that can be easily tested and modified in the future.
This is all incredibly important when considering the Dependency Inversion Principle. By applying DIP, we consciously design our dependencies in such a way that they depend on abstractions rather than concrete classes. This means that changing one component does not negatively impact others, reflecting the philosophy of SRP.
Another principle worth comparing with DIP is the open-closed (Open/Closed Principle, OCP) principle. OCP advises that modules should be open for extension but closed for modification. Now, imagine you have your favorite cake recipe. The first time you bake it, it's delicious, and you're proud. But instead of completely changing this recipe every time, it's better to add new flavors or ingredients to maintain the original taste and structure.
In the context of DIP, this principle suggests that we use interfaces and abstractions to add new functionalities without modifying the existing logic in the code—here, too, it plays into modularity and flexibility.
Next, let’s look at the Liskov (Liskov Substitution Principle, LSP) and interface segregation (Interface Segregation Principle, ISP) principles. LSP tells us that objects of derived classes should be able to replace objects of base classes without altering the behavior of the program. This means that our code should be structured to comply with LSP’s assumptions—otherwise, we risk our program falling apart like a poorly oiled mechanism.
Meanwhile, ISP advises us to design interfaces to be small and concise. You want the class implementing the interface to know only what it needs and not be forced to implement methods it will never use. When well-designed, they work together with DIP, enabling easy addition of new features and great freedom in creating new classes.
At the same time, the SOLID principles maintain an ideal balance with each other. When one is violated, the others may not be able to achieve forward-looking goals regarding behavior and quality in our software.
It’s worth noting that all of them are like moves on the board—well-planned, yet flexible, allowing programmers to enjoy a strategic game. Moreover, each principle aims to minimize complexity through decomposition and isolating dependencies, making our applications more reliable and future-proof.
All of these principles are like a well-organized orchestra, where each instrument has its role, and when they play together, they achieve harmony. By using the Dependency Inversion Principle as a focal point, we can create an application that is not only easy to manage but also ready for all kinds of changes and challenges. By understanding how these principles interact, a completely new dimension of programming opens up before us.
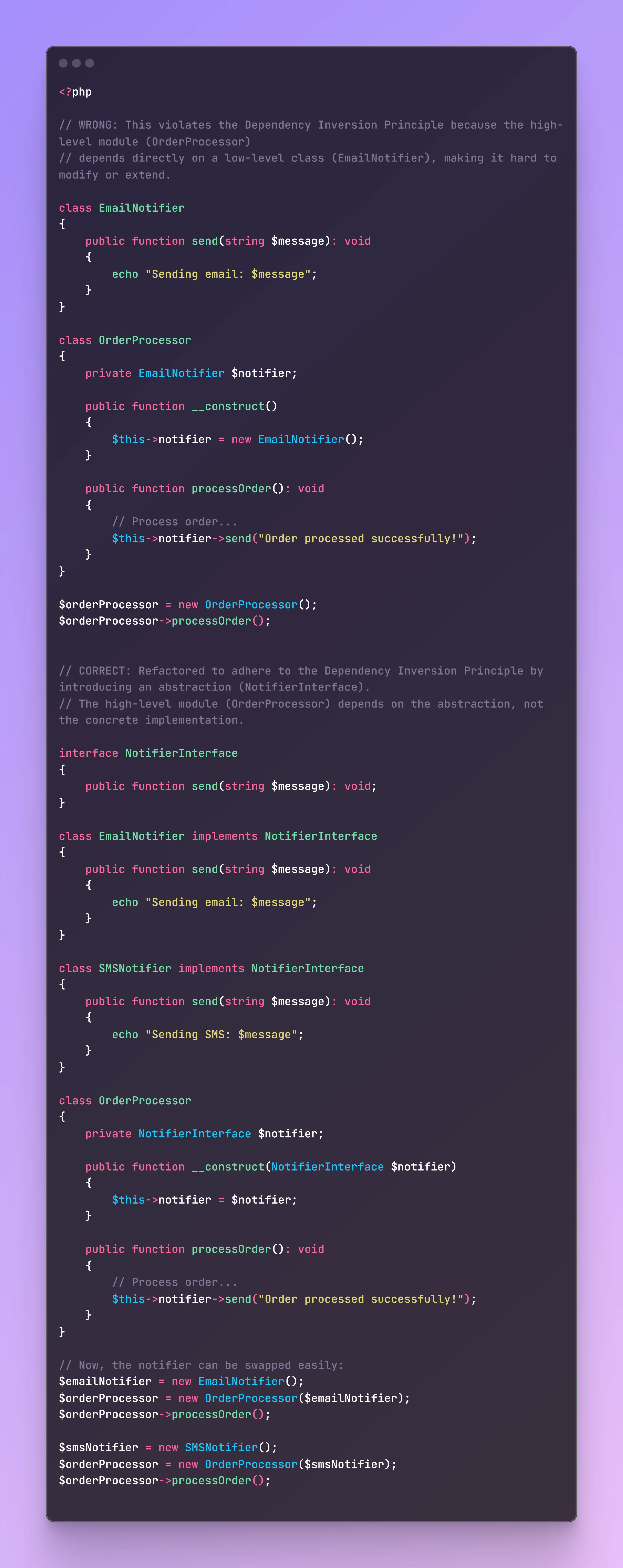
As we approach the end of our journey through the intricacies of the Dependency Inversion Principle, it is worth pausing for a moment to closely examine what we have discovered at the generous thresholds of SOLID. This principle, though sounding like complicated magic to some, proves to be an extremely practical technique that significantly enhances the quality of our code. Like a true composer who blends different instruments into a harmonious symphony, the Dependency Inversion Principle allows us to focus on the key elements of a system, arranging them into a coherent whole.
Strengthening the weak points of code is a topic familiar to anyone. In code, just as in life, the goal is to minimize risk and avoid pitfalls. When we break our dependencies and make them loosely coupled, we open doors to greater flexibility and easier project management. This can be compared to the infrastructure of a bridge – the better it is designed, the more it can withstand various loads. As you go through the SOLID principles, you must have felt how you distribute those loads, gaining better control over what is really happening in your code.
Design Standards
When we talk about design standards, the Dependency Inversion Principle aims particularly to ensure that higher levels of code are not unpleasantly tied to lower-level details. What does this exactly mean? An example can be related to the construction of houses.
- We want the foundations to be solid and well-designed, while at the same time, the foundations should not dictate the design of the whole.
- Key in this matter is to safeguard the infrastructure at the upper level, making changes and modifications less invasive.
With inverted dependencies, we become more aware creators of code, and our structure takes on a new expression. By choosing interfaces as boundaries between the components of our application, we become like architects tinkering with a seaside villa, where every inch of space counts in every regard.
The overarching classes can be strengthened, and low-level components can find themselves in new roles, while their original functionality remains unchanged.
Philosophy of Dependency Inversion
A proper understanding of the Dependency Inversion Principle is not just a technique but also a philosophy. As you well know, programming is an art in which skills require continuous development and preparation for sudden twists. Enjoying this experience, we face new challenges, making us better programmers.
The increase in efficiency we can achieve through the implementation of this principle represents the greatest gain. Our ability to adapt to the changing market requirements and the ease of adapting to new software versions ensures us long-term success in the field of programming.
Summary
In summary, the Dependency Inversion Principle, despite its complexity, is a valuable ally in the fight for high-quality code. We encourage you to delve into this topic and implement it in practical projects. We dedicate ourselves to the pleasure of creating code as diverse as architectural plans, while being as flexible as the best rubber band, capable of withstanding any test arising from changes in client requirements.
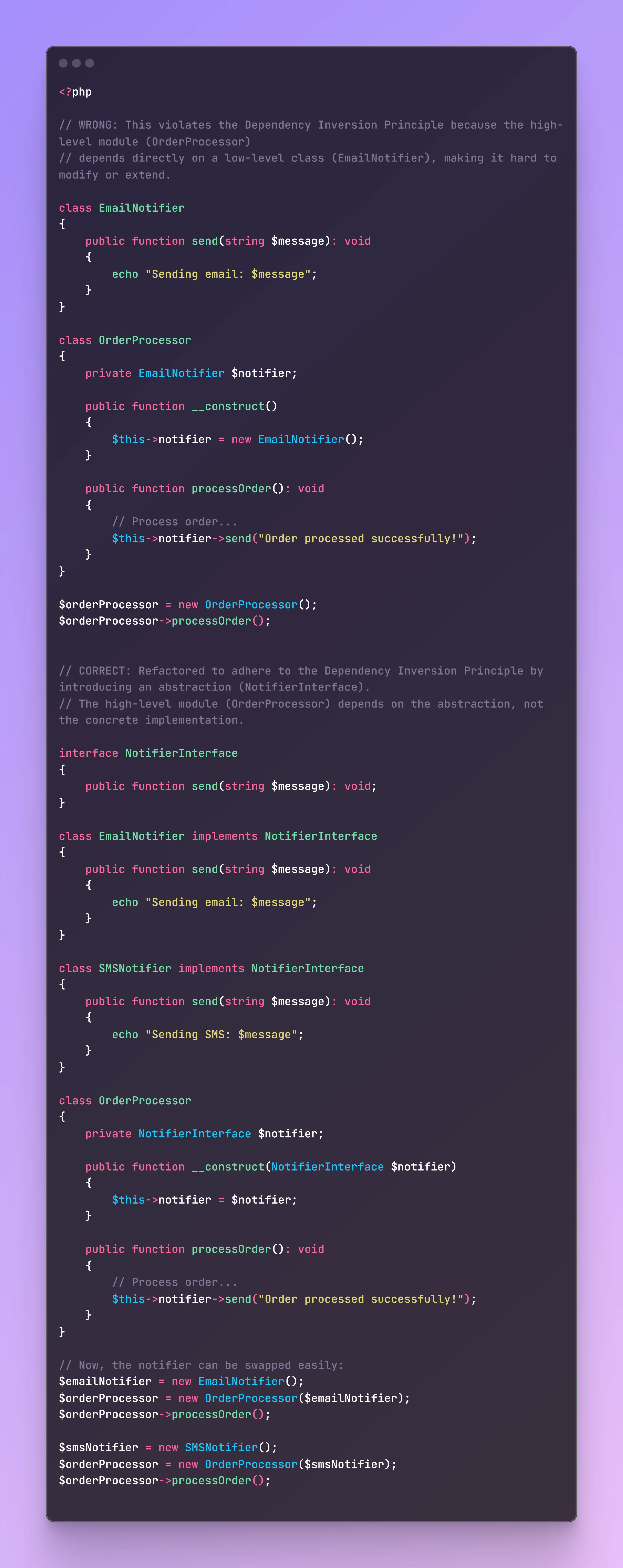